基于哈希表的信息系统 定义一个学生类Student,学生信息包括学号,姓名,年龄,成绩。 学号和姓名均为字符串类型,年龄为整数类型。 设计一个班级类Clazz,它包括一组学生,并采用哈希表来存储这组学生。哈希表使用学生学号作为Key,学生对象作为Value。 在Clazz类中实现以下功能: 添加:添加一个学生对象; 查找:能够根据学号找到该学生对象; 修改:修改根据学号找到的学生对象的信息; 删除:删除指定学号的学生对象; 打印: 能够打印单个学生信息; 能够按学号顺序打印所有学生信息,每个学生信息占一行; 能够根据成绩从高到底打印所有学生信息,每个学生信息占一行。 在main中设计一个流程,打印一个菜单,并根据菜单输入相应的选项进行学生的添加、查找、修改、删除和打印等。
时间: 2023-05-30 16:04:01 浏览: 55
下面是基于哈希表的信息系统的代码实现:
// 定义学生类
class Student {
public:
Student(string id, string name, int age, double score) {
this->id = id;
this->name = name;
this->age = age;
this->score = score;
}
string getId() {
return id;
}
string getName() {
return name;
}
int getAge() {
return age;
}
double getScore() {
return score;
}
void setName(string name) {
this->name = name;
}
void setAge(int age) {
this->age = age;
}
void setScore(double score) {
this->score = score;
}
private:
string id;
string name;
int age;
double score;
};
// 定义班级类
class Clazz {
public:
Clazz() {}
void addStudent(Student* student) {
students[student->getId()] = student;
}
Student* findStudent(string id) {
if (students.count(id) > 0) {
return students[id];
} else {
return NULL;
}
}
void modifyStudent(string id, string name, int age, double score) {
if (students.count(id) > 0) {
Student* student = students[id];
student->setName(name);
student->setAge(age);
student->setScore(score);
}
}
void deleteStudent(string id) {
if (students.count(id) > 0) {
students.erase(id);
}
}
void printStudent(Student* student) {
cout << "学号:" << student->getId() << ",姓名:" << student->getName() << ",年龄:" << student->getAge() << ",成绩:" << student->getScore() << endl;
}
void printAllStudentsById() {
map<string, Student*>::iterator it;
for (it = students.begin(); it != students.end(); it++) {
printStudent(it->second);
}
}
bool compareByScore(Student* a, Student* b) {
return a->getScore() > b->getScore();
}
void printAllStudentsByScore() {
vector<Student*> studentList;
map<string, Student*>::iterator it;
for (it = students.begin(); it != students.end(); it++) {
studentList.push_back(it->second);
}
sort(studentList.begin(), studentList.end(), compareByScore);
for (int i = 0; i < studentList.size(); i++) {
printStudent(studentList[i]);
}
}
private:
map<string, Student*> students;
};
// 主函数
int main() {
Clazz* clazz = new Clazz();
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 添加学生" << endl;
cout << "2. 查找学生" << endl;
cout << "3. 修改学生" << endl;
cout << "4. 删除学生" << endl;
cout << "5. 按学号顺序打印所有学生信息" << endl;
cout << "6. 按成绩从高到低打印所有学生信息" << endl;
cout << "0. 退出" << endl;
int option;
cin >> option;
if (option == 0) {
break;
} else if (option == 1) {
cout << "请输入学生学号、姓名、年龄、成绩(以空格分隔):" << endl;
string id, name;
int age;
double score;
cin >> id >> name >> age >> score;
Student* student = new Student(id, name, age, score);
clazz->addStudent(student);
cout << "添加成功" << endl;
} else if (option == 2) {
cout << "请输入学生学号:" << endl;
string id;
cin >> id;
Student* student = clazz->findStudent(id);
if (student != NULL) {
clazz->printStudent(student);
} else {
cout << "未找到该学生" << endl;
}
} else if (option == 3) {
cout << "请输入学生学号、姓名、年龄、成绩(以空格分隔):" << endl;
string id, name;
int age;
double score;
cin >> id >> name >> age >> score;
clazz->modifyStudent(id, name, age, score);
cout << "修改成功" << endl;
} else if (option == 4) {
cout << "请输入学生学号:" << endl;
string id;
cin >> id;
clazz->deleteStudent(id);
cout << "删除成功" << endl;
} else if (option == 5) {
clazz->printAllStudentsById();
} else if (option == 6) {
clazz->printAllStudentsByScore();
} else {
cout << "无效操作,请重新输入" << endl;
}
}
return 0;
}
相关推荐
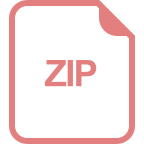
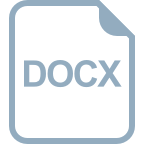
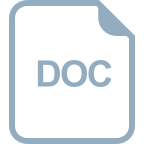














