1. 加载训练好的train.h5模型(5) 2. 使用训练后的模型及 "model.predict()"函数对测试集test_set进行验证,并按照要求指出测试集中某张图片预测结果的含义(10)
时间: 2024-12-17 15:18:18 浏览: 11
1. 要加载已经训练好的H5格式模型(如`train.h5`),在Keras中通常需要使用`load_model`函数。首先,确保你导入了所需的库,比如:
```python
import keras
from keras.models import load_model
# 加载模型
model = load_model('train.h5')
```
这个`model`变量现在包含了训练好的权重,可以用于预测。
2. 对于`model.predict()`函数,它接收一个数据集作为输入,并返回每个样本的预测结果。假设`test_set`是一个包含图像数组的数据集,例如`numpy`数组,你可以按如下步骤进行:
```python
# 假设test_set有两部分:测试图像数据(test_images)和对应标签(test_labels)
predictions = model.predict(test_images)
# 预测通常是概率值,对于分类任务,最大概率对应的索引就是模型预测的类别
predicted_classes = np.argmax(predictions, axis=1)
# 对于某个特定图片(假设其索引为image_index)
image_prediction = predicted_classes[image_index]
```
这里的`image_prediction`是一个整数,代表该图片被预测属于数据集中哪个类别的概率最高的类别。如果这是一个二分类问题,0可能表示类别A,1表示类别B;如果是多分类,数字则对应类别编号。
相关问题
请问这段代码如何给目标函数加入约束:8-x[0]-2*x[1]>=0:import numpy as np import tensorflow as tf from tensorflow.keras import layers import matplotlib.pyplot as plt # 定义目标函数 def objective_function(x): return x[0]-x[1]-x[2]-x[0]*x[2]+x[0]*x[3]+x[1]*x[2]-x[1]*x[3] # 生成训练数据 num_samples = 1000 X_train = np.random.random((num_samples, 4)) y_train = np.array([objective_function(x) for x in X_train]) # 划分训练集和验证集 split_ratio = 0.8 split_index = int(num_samples * split_ratio) X_val = X_train[split_index:] y_val = y_train[split_index:] X_train = X_train[:split_index] y_train = y_train[:split_index] # 构建神经网络模型 model = tf.keras.Sequential([ layers.Dense(32, activation='relu', input_shape=(4,)), layers.Dense(32, activation='relu'), layers.Dense(1) ]) # 编译模型 model.compile(tf.keras.optimizers.Adam(), loss='mean_squared_error') # 设置保存模型的路径 model_path = "model.h5" # 训练模型 history = model.fit(X_train, y_train, validation_data=(X_val, y_val), epochs=100, batch_size=32) # 保存模型 model.save(model_path) print("模型已保存") # 加载模型 loaded_model = tf.keras.models.load_model(model_path) print("模型已加载") # 使用模型预测最小值 a =np.random.uniform(0,5,size=4) X_test=np.array([a]) y_pred = loaded_model.predict(X_test) print("随机取样点",X_test) print("最小值:", y_pred[0]) # 可视化训练过程 plt.plot(history.history['loss'], label='train_loss') plt.plot(history.history['val_loss'], label='val_loss') plt.xlabel('Epoch') plt.ylabel('Loss') plt.legend() plt.show()
要给目标函数加入约束,可以在训练数据生成的过程中进行约束条件的判断,并将不满足约束条件的样本剔除或进行修正。
在这段代码中,约束条件为8 - x[0] - 2*x[1] >= 0。可以在生成训练数据的步骤中添加对该约束条件的判断,如下所示:
```python
# 生成训练数据
num_samples = 1000
X_train = []
y_train = []
while len(X_train) < num_samples:
x = np.random.random((4,))
if 8 - x[0] - 2*x[1] >= 0:
X_train.append(x)
y_train.append(objective_function(x))
X_train = np.array(X_train)
y_train = np.array(y_train)
```
这样,生成的训练数据集就满足约束条件了。之后的训练、验证和预测过程可以保持不变。
注意:在实际应用中,可能需要根据具体的约束条件进行相应的修改。
这段代码应该如何修改将训练出来的深度神经网络与优化算法相结合求出目标函数最小值:# 构建神经网络模型 model = tf.keras.Sequential([ layers.Dense(64, activation='relu', input_shape=(4,)), layers.Dense(64, activation='relu'), layers.Dense(32, activation='relu'), layers.Dense(32, activation='relu'), layers.Dense(16, activation='relu'), layers.Dense(8, activation='relu'), layers.Dense(4, activation='relu'), layers.Dense(1) ]) # 编译模型 model.compile(tf.keras.optimizers.Adam(), loss='mean_squared_error') # 设置保存模型的路径 model_path = "model.h5" # 训练模型 history = model.fit(X_train, y_train, validation_data=(X_val, y_val), epochs=50, batch_size=32) # 保存模型 model.save(model_path) print("模型已保存") # 加载模型 loaded_model = tf.keras.models.load_model(model_path) print("模型已加载") # 随机生成满足约束条件的样本并使用模型预测最小值 a = np.random.uniform(0,5,size=4) while not ((8-a[0]-2*a[1] and 12 - 4*a[0] - a[1] >= 0) and (12 - 3*a[0] - 4*a[1] >= 0) and (8 - 2*a[2] - a[3] >= 0) and (8 - a[2] - 2*a[3] >= 0) and (5 - a[2] - a[3] >= 0) and (0 <= a[0]) and (a[0] <= 5) and (0 <= a[1]) and (a[1] <= 5) and (0 <= a[2]) and (a[2] <= 5) and (0 <= a[3]) and (a[3] <= 5)): a = np.random.uniform(0,5,size=4) X_test = np.array([a]) y_pred = model.predict(X_test) print("随机取样点:", X_test) print("最小值:", y_pred[0][0])
要将训练出来的深度神经网络与优化算法相结合,可以使用深度神经网络来近似解决问题,并结合优化算法进行迭代优化。
以下是修改后的代码示例:
```python
import tensorflow as tf
import numpy as np
import cvxpy as cp
# 构建神经网络模型
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(4,)),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(32, activation='relu'),
tf.keras.layers.Dense(32, activation='relu'),
tf.keras.layers.Dense(16, activation='relu'),
tf.keras.layers.Dense(8, activation='relu'),
tf.keras.layers.Dense(4, activation='relu'),
tf.keras.layers.Dense(1)
])
# 编译模型
model.compile(optimizer=tf.keras.optimizers.Adam(), loss='mean_squared_error')
# 设置保存模型的路径
model_path = "model.h5"
# 训练模型
history = model.fit(X_train, y_train, validation_data=(X_val, y_val), epochs=50, batch_size=32)
# 保存模型
model.save(model_path)
print("模型已保存")
# 加载模型
loaded_model = tf.keras.models.load_model(model_path)
print("模型已加载")
# 定义约束条件函数
def constraints(x):
return [
8 - x[0] - 2*x[1] >= 0,
12 - 4*x[0] - x[1] >= 0,
12 - 3*x[0] - 4*x[1] >= 0,
8 - 2*x[2] - x[3] >= 0,
8 - x[2] - 2*x[3] >= 0,
5 - x[2] - x[3] >= 0,
x >= 0,
x <= 5
]
# 使用优化算法迭代优化
x = cp.Variable(4)
constraints = constraints(x)
objective = cp.Minimize(model(x))
problem = cp.Problem(objective, constraints)
problem.solve()
# 输出结果
print("最小化函数值:", problem.value)
print("最优解:", x.value)
```
在这个示例中,我们首先定义了一个深度神经网络模型,并使用训练数据进行训练,并保存模型。然后,我们使用加载的模型来定义目标函数,并结合约束条件构建优化问题。最后,使用优化算法进行迭代优化,求解最小值并输出结果。
请注意,这里只是给出了一个简单的示例,实际应用中可能需要根据具体情况进行相应的修改和调整。此外,对于复杂的问题,可能需要使用更高级的优化算法来求解。
阅读全文
相关推荐
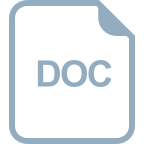
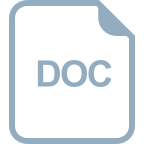
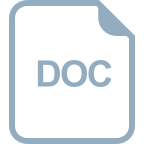
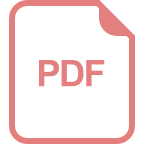












