用C语言写一个 字符双向循环链表,实现插入手动输入的字符
时间: 2023-04-07 22:00:56 浏览: 129
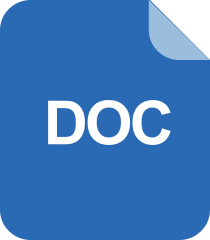
双向循环链表-C语言实现.doc
好的,这是一个技术问题,我可以回答。以下是用C语言实现字符双向循环链表的代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
char data;
struct node *prev;
struct node *next;
} Node;
Node *create_node(char data) {
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->data = data;
new_node->prev = NULL;
new_node->next = NULL;
return new_node;
}
void insert_node(Node **head, char data) {
Node *new_node = create_node(data);
if (*head == NULL) {
*head = new_node;
(*head)->prev = *head;
(*head)->next = *head;
} else {
new_node->prev = (*head)->prev;
new_node->next = *head;
(*head)->prev->next = new_node;
(*head)->prev = new_node;
}
}
void print_list(Node *head) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
Node *current = head;
do {
printf("%c ", current->data);
current = current->next;
} while (current != head);
printf("\n");
}
int main() {
Node *head = NULL;
char input;
printf("Please enter characters to insert into the list (enter '.' to stop):\n");
do {
scanf(" %c", &input);
if (input != '.') {
insert_node(&head, input);
}
} while (input != '.');
printf("The list is:\n");
print_list(head);
return 0;
}
```
这个程序可以让用户手动输入字符,并将它们插入到双向循环链表中。最后,程序会输出整个链表中的字符。
阅读全文
相关推荐
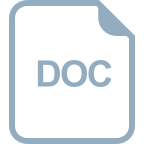
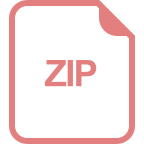
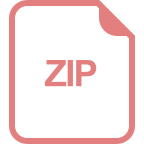
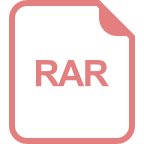
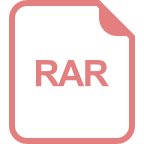
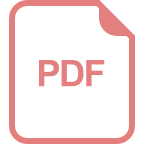
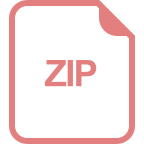
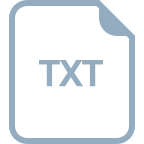
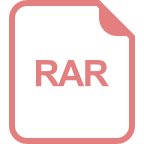
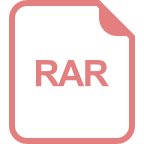
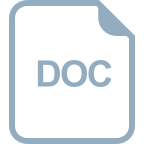
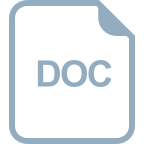
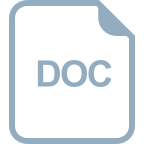
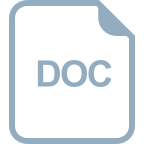