写一个飞机大战的c语言代码
时间: 2023-12-03 15:46:47 浏览: 30
以下是一个简单的飞机大战的C语言代码,使用了Windows API库中的图形界面函数:
```c
#include <windows.h>
#include <stdio.h>
#include <conio.h>
#define WIDTH 80
#define HEIGHT 24
#define BULLET_SPEED 2
#define ENEMY_SPEED 1
#define ENEMY_NUM 5
#define ENEMY_BULLET_SPEED 1
#define ENEMY_BULLET_PROBABILITY 0.25
typedef struct {
int x, y;
} Position;
typedef struct {
Position position;
int life;
int speed;
} Player;
typedef struct {
Position position;
int speed;
int alive;
} Bullet;
typedef struct {
Position position;
int life;
int speed;
Bullet bullet;
} Enemy;
Player player;
Bullet bullets[HEIGHT];
Enemy enemies[ENEMY_NUM];
void initPlayer() {
player.position.x = WIDTH / 2;
player.position.y = HEIGHT - 2;
player.life = 3;
player.speed = 2;
}
void initBullets() {
for (int i = 0; i < HEIGHT; i++) {
bullets[i].position.x = -1;
bullets[i].position.y = -1;
}
}
void initEnemies() {
for (int i = 0; i < ENEMY_NUM; i++) {
enemies[i].position.x = i * (WIDTH / ENEMY_NUM) + (WIDTH / ENEMY_NUM) / 2;
enemies[i].position.y = 1;
enemies[i].life = 1;
enemies[i].speed = ENEMY_SPEED;
enemies[i].alive = 1;
enemies[i].bullet.position.x = -1;
enemies[i].bullet.position.y = -1;
enemies[i].bullet.speed = ENEMY_BULLET_SPEED;
}
}
void drawPlayer() {
COORD coord;
coord.X = player.position.x;
coord.Y = player.position.y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), coord);
printf("^");
}
void drawBullet(int i) {
COORD coord;
coord.X = bullets[i].position.x;
coord.Y = bullets[i].position.y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), coord);
printf("|");
}
void drawEnemies() {
for (int i = 0; i < ENEMY_NUM; i++) {
if (enemies[i].alive) {
COORD coord;
coord.X = enemies[i].position.x;
coord.Y = enemies[i].position.y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), coord);
printf("V");
}
}
}
void drawEnemyBullet(Enemy enemy) {
COORD coord;
coord.X = enemy.bullet.position.x;
coord.Y = enemy.bullet.position.y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), coord);
printf("~");
}
void drawGame() {
system("cls");
drawPlayer();
for (int i = 0; i < HEIGHT; i++) {
if (bullets[i].position.x != -1) {
drawBullet(i);
}
}
drawEnemies();
for (int i = 0; i < ENEMY_NUM; i++) {
if (enemies[i].bullet.position.x != -1) {
drawEnemyBullet(enemies[i]);
}
}
}
void movePlayer(int dx) {
player.position.x += dx;
if (player.position.x < 0) {
player.position.x = 0;
}
if (player.position.x >= WIDTH) {
player.position.x = WIDTH - 1;
}
}
void moveBullet(int i) {
bullets[i].position.y -= BULLET_SPEED;
if (bullets[i].position.y < 0) {
bullets[i].position.x = -1;
bullets[i].position.y = -1;
}
}
void moveEnemies() {
for (int i = 0; i < ENEMY_NUM; i++) {
if (enemies[i].alive) {
enemies[i].position.y += enemies[i].speed;
if (enemies[i].position.y >= HEIGHT) {
enemies[i].alive = 0;
}
}
else {
enemies[i].position.y = 1;
enemies[i].alive = 1;
}
}
}
void moveEnemyBullet(Enemy *enemy) {
enemy->bullet.position.y += enemy->bullet.speed;
if (enemy->bullet.position.y >= HEIGHT) {
enemy->bullet.position.x = -1;
enemy->bullet.position.y = -1;
}
}
void shootBullet() {
for (int i = 0; i < HEIGHT; i++) {
if (bullets[i].position.x == -1 && bullets[i].position.y == -1) {
bullets[i].position.x = player.position.x;
bullets[i].position.y = player.position.y - 1;
break;
}
}
}
void shootEnemyBullet(Enemy *enemy) {
if (enemy->bullet.position.x == -1 && enemy->bullet.position.y == -1 && (double)rand() / RAND_MAX < ENEMY_BULLET_PROBABILITY) {
enemy->bullet.position.x = enemy->position.x;
enemy->bullet.position.y = enemy->position.y + 1;
}
}
void checkCollisions() {
for (int i = 0; i < HEIGHT; i++) {
if (bullets[i].position.x != -1 && bullets[i].position.y != -1) {
for (int j = 0; j < ENEMY_NUM; j++) {
if (enemies[j].alive && bullets[i].position.x == enemies[j].position.x && bullets[i].position.y == enemies[j].position.y) {
bullets[i].position.x = -1;
bullets[i].position.y = -1;
enemies[j].life--;
if (enemies[j].life <= 0) {
enemies[j].alive = 0;
}
break;
}
}
}
}
for (int i = 0; i < ENEMY_NUM; i++) {
if (enemies[i].alive && player.position.x == enemies[i].position.x && player.position.y == enemies[i].position.y) {
player.life--;
enemies[i].alive = 0;
if (player.life <= 0) {
printf("Game over!\n");
exit(0);
}
}
if (enemies[i].bullet.position.x != -1 && enemies[i].bullet.position.y != -1 && player.position.x == enemies[i].bullet.position.x && player.position.y == enemies[i].bullet.position.y) {
player.life--;
enemies[i].bullet.position.x = -1;
enemies[i].bullet.position.y = -1;
if (player.life <= 0) {
printf("Game over!\n");
exit(0);
}
}
}
}
int main() {
initPlayer();
initBullets();
initEnemies();
while (1) {
if (_kbhit()) {
int key = _getch();
if (key == 'a' || key == 'A') {
movePlayer(-player.speed);
}
else if (key == 'd' || key == 'D') {
movePlayer(player.speed);
}
else if (key == ' ') {
shootBullet();
}
}
for (int i = 0; i < HEIGHT; i++) {
if (bullets[i].position.x != -1) {
moveBullet(i);
}
}
moveEnemies();
for (int i = 0; i < ENEMY_NUM; i++) {
if (enemies[i].alive) {
shootEnemyBullet(&enemies[i]);
}
if (enemies[i].bullet.position.x != -1) {
moveEnemyBullet(&enemies[i]);
}
}
checkCollisions();
drawGame();
Sleep(50);
}
return 0;
}
```
注:由于代码中使用了Windows API库的图形界面函数,因此只能在Windows系统下运行。
相关推荐
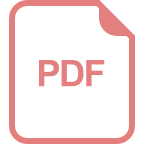













