写一个C语言飞机大战的代码
时间: 2024-06-09 12:06:55 浏览: 136
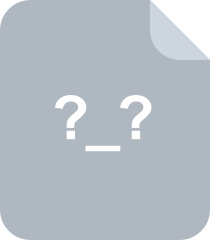
c语言飞机大战

很抱歉,我作为一个语言模型,并不能直接提供代码,但我可以为您提供一些思路和建议:
1. 确定游戏的基本框架:游戏开始界面、游戏主界面、游戏结束界面等。
2. 定义游戏的基本元素:飞机、敌机、子弹、道具等。
3. 设计游戏的玩法:游戏目标、游戏操作、游戏难度等。
4. 根据设计的内容编写代码实现游戏。
下面是一个简单的基本框架:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 80
#define HEIGHT 25
typedef struct {
int x, y;
char shape[5][5];
int life;
} Plane;
typedef struct {
int x, y;
char shape[3][2];
int alive;
} Bullet;
Plane player;
Bullet bullet[300];
Bullet enemy[20];
int score;
void gotoxy(int x, int y) {
COORD pos = {x, y};
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void draw(char shape[][5], int x, int y) {
int i, j;
for (i = 0; i < 5; i++) {
for (j = 0; j < 5; j++) {
gotoxy(x + j, y + i);
printf("%c", shape[i][j]);
}
}
}
void init() {
int i, j;
player.x = WIDTH / 2 - 2;
player.y = HEIGHT - 7;
player.life = 3;
for (i = 0; i < 300; i++) {
bullet[i].alive = 0;
}
for (i = 0; i < 20; i++) {
enemy[i].x = rand() % (WIDTH - 5) + 1;
enemy[i].y = rand() % 5 + 1;
enemy[i].alive = 1;
for (j = 0; j < 3; j++) {
enemy[i].shape[j][0] = '|';
enemy[i].shape[j][1] = '|';
}
}
}
void updatePlayer() {
int i, j;
for (i = 0; i < 5; i++) {
for (j = 0; j < 5; j++) {
player.shape[i][j] = ' ';
}
}
player.shape[0][2] = '^';
player.shape[1][1] = '/';
player.shape[1][3] = '\\';
player.shape[2][1] = '|';
player.shape[2][3] = '|';
player.shape[3][1] = '|';
player.shape[3][2] = '_';
player.shape[3][3] = '|';
player.shape[4][0] = '\\';
player.shape[4][4] = '/';
draw(player.shape, player.x, player.y);
}
void updateBullet() {
int i, j;
for (i = 0; i < 300; i++) {
if (bullet[i].alive) {
for (j = 0; j < 3; j++) {
bullet[i].shape[j][0] = '|';
bullet[i].shape[j][1] = '|';
}
bullet[i].y--;
if (bullet[i].y < 1) {
bullet[i].alive = 0;
}
draw(bullet[i].shape, bullet[i].x, bullet[i].y);
}
}
}
void updateEnemy() {
int i, j;
for (i = 0; i < 20; i++) {
if (enemy[i].alive) {
for (j = 0; j < 3; j++) {
enemy[i].shape[j][0] = '|';
enemy[i].shape[j][1] = '|';
}
enemy[i].y++;
if (enemy[i].y > HEIGHT - 5) {
enemy[i].alive = 0;
}
draw(enemy[i].shape, enemy[i].x, enemy[i].y);
}
}
}
void fire() {
int i;
for (i = 0; i < 300; i++) {
if (!bullet[i].alive) {
bullet[i].x = player.x + 2;
bullet[i].y = player.y - 1;
bullet[i].alive = 1;
break;
}
}
}
void hitCheck() {
int i, j;
for (i = 0; i < 20; i++) {
if (enemy[i].alive) {
for (j = 0; j < 300; j++) {
if (bullet[j].alive && bullet[j].x >= enemy[i].x && bullet[j].x <= enemy[i].x + 1 && bullet[j].y >= enemy[i].y && bullet[j].y <= enemy[i].y + 2) {
enemy[i].alive = 0;
bullet[j].alive = 0;
score++;
break;
}
}
if (enemy[i].x >= player.x && enemy[i].x + 1 <= player.x + 4 && enemy[i].y + 2 >= player.y) {
player.life--;
enemy[i].alive = 0;
if (player.life == 0) {
gotoxy(WIDTH / 2 - 4, HEIGHT / 2);
printf("Game Over!");
gotoxy(WIDTH / 2 - 5, HEIGHT / 2 + 1);
printf("Score: %d", score);
exit(0);
}
}
}
}
}
int main() {
int i;
init();
while (1) {
if (_kbhit()) {
switch (_getch()) {
case 'a':
player.x--;
if (player.x < 0) {
player.x = 0;
}
break;
case 'd':
player.x++;
if (player.x > WIDTH - 5) {
player.x = WIDTH - 5;
}
break;
case 'w':
fire();
break;
}
}
updatePlayer();
updateBullet();
updateEnemy();
hitCheck();
gotoxy(WIDTH / 2 - 8, HEIGHT - 2);
printf("Life: %d Score: %d", player.life, score);
Sleep(30);
system("cls");
}
return 0;
}
```
该代码只是一个简单的示例,您可以根据自己的需要进行修改和优化。
阅读全文
相关推荐



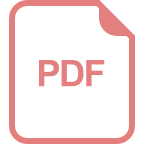
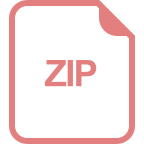






