windows系统下C语言飞机大战代码
时间: 2024-02-18 21:34:28 浏览: 88
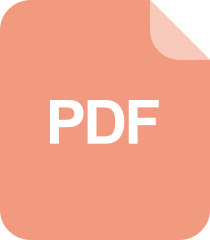
c语言飞机大战源代码.pdf

以下是一个简单的 Windows 系统下的 C 语言飞机大战代码,使用了 WinAPI 库。代码中实现了一个简单的游戏界面和飞机控制,具体细节可以根据需要进行修改和完善。
```c
#include <windows.h>
#include <stdlib.h>
#include <time.h>
#define MAX_ENEMIES 10
#define ENEMY_SPEED 3
#define ENEMY_WIDTH 50
#define ENEMY_HEIGHT 50
#define BULLET_SPEED 8
#define BULLET_WIDTH 5
#define BULLET_HEIGHT 10
#define PLAYER_SPEED 5
#define PLAYER_WIDTH 50
#define PLAYER_HEIGHT 50
static int score = 0;
static int game_over = 0;
typedef struct {
int x;
int y;
int alive;
} enemy_t;
typedef struct {
int x;
int y;
int alive;
} bullet_t;
typedef struct {
int x;
int y;
} player_t;
static enemy_t enemies[MAX_ENEMIES];
static bullet_t bullets[MAX_ENEMIES];
static player_t player;
void init_game() {
int i;
for (i = 0; i < MAX_ENEMIES; i++) {
enemies[i].x = rand() % (600 - ENEMY_WIDTH);
enemies[i].y = rand() % 1000 - 1000;
enemies[i].alive = 1;
}
for (i = 0; i < MAX_ENEMIES; i++) {
bullets[i].x = -1;
bullets[i].y = -1;
bullets[i].alive = 0;
}
player.x = 275;
player.y = 700;
}
void draw_enemy(HDC hdc, int x, int y) {
HBRUSH brush = CreateSolidBrush(RGB(255, 0, 0));
HGDIOBJ old_brush = SelectObject(hdc, brush);
Rectangle(hdc, x, y, x + ENEMY_WIDTH, y + ENEMY_HEIGHT);
SelectObject(hdc, old_brush);
DeleteObject(brush);
}
void draw_bullet(HDC hdc, int x, int y) {
HBRUSH brush = CreateSolidBrush(RGB(0, 0, 255));
HGDIOBJ old_brush = SelectObject(hdc, brush);
Rectangle(hdc, x, y, x + BULLET_WIDTH, y + BULLET_HEIGHT);
SelectObject(hdc, old_brush);
DeleteObject(brush);
}
void draw_player(HDC hdc, int x, int y) {
HBRUSH brush = CreateSolidBrush(RGB(0, 255, 0));
HGDIOBJ old_brush = SelectObject(hdc, brush);
Rectangle(hdc, x, y, x + PLAYER_WIDTH, y + PLAYER_HEIGHT);
SelectObject(hdc, old_brush);
DeleteObject(brush);
}
void update_game(HWND hwnd) {
int i, j;
if (game_over) {
MessageBox(hwnd, "Game over", "Game over", MB_OK);
init_game();
score = 0;
game_over = 0;
}
for (i = 0; i < MAX_ENEMIES; i++) {
if (enemies[i].alive) {
enemies[i].y += ENEMY_SPEED;
if (enemies[i].y > 800) {
enemies[i].x = rand() % (600 - ENEMY_WIDTH);
enemies[i].y = rand() % 1000 - 1000;
enemies[i].alive = 1;
}
for (j = 0; j < MAX_ENEMIES; j++) {
if (bullets[j].alive && bullets[j].x > enemies[i].x && bullets[j].x < enemies[i].x + ENEMY_WIDTH && bullets[j].y > enemies[i].y && bullets[j].y < enemies[i].y + ENEMY_HEIGHT) {
enemies[i].alive = 0;
bullets[j].alive = 0;
score++;
break;
}
}
if (player.x > enemies[i].x && player.x < enemies[i].x + ENEMY_WIDTH && player.y > enemies[i].y && player.y < enemies[i].y + ENEMY_HEIGHT) {
game_over = 1;
}
}
}
for (i = 0; i < MAX_ENEMIES; i++) {
if (bullets[i].alive) {
bullets[i].y -= BULLET_SPEED;
if (bullets[i].y < 0) {
bullets[i].alive = 0;
}
}
}
}
void draw_game(HWND hwnd, HDC hdc) {
int i;
char score_str[100];
sprintf(score_str, "Score: %d", score);
TextOut(hdc, 10, 10, score_str, strlen(score_str));
for (i = 0; i < MAX_ENEMIES; i++) {
if (enemies[i].alive) {
draw_enemy(hdc, enemies[i].x, enemies[i].y);
}
}
for (i = 0; i < MAX_ENEMIES; i++) {
if (bullets[i].alive) {
draw_bullet(hdc, bullets[i].x, bullets[i].y);
}
}
draw_player(hdc, player.x, player.y);
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam) {
HDC hdc;
PAINTSTRUCT ps;
switch (msg) {
case WM_CREATE:
srand(time(NULL));
init_game();
SetTimer(hwnd, 1, 30, NULL);
break;
case WM_TIMER:
update_game(hwnd);
InvalidateRect(hwnd, NULL, FALSE);
break;
case WM_PAINT:
hdc = BeginPaint(hwnd, &ps);
draw_game(hwnd, hdc);
EndPaint(hwnd, &ps);
break;
case WM_KEYDOWN:
switch (wParam) {
case VK_LEFT:
if (player.x > 0) {
player.x -= PLAYER_SPEED;
}
break;
case VK_RIGHT:
if (player.x < 550) {
player.x += PLAYER_SPEED;
}
break;
case VK_SPACE:
for (int i = 0; i < MAX_ENEMIES; i++) {
if (!bullets[i].alive) {
bullets[i].x = player.x + PLAYER_WIDTH / 2 - BULLET_WIDTH / 2;
bullets[i].y = player.y - BULLET_HEIGHT;
bullets[i].alive = 1;
break;
}
}
break;
default:
break;
}
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, msg, wParam, lParam);
}
return 0;
}
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) {
WNDCLASSEX wc;
HWND hwnd;
MSG msg;
ZeroMemory(&wc, sizeof(WNDCLASSEX));
wc.cbSize = sizeof(WNDCLASSEX);
wc.style = CS_HREDRAW | CS_VREDRAW;
wc.lpfnWndProc = WndProc;
wc.hInstance = hInstance;
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.lpszClassName = "MainWindowClass";
RegisterClassEx(&wc);
hwnd = CreateWindowEx(WS_EX_CLIENTEDGE, "MainWindowClass", "飞机大战", WS_OVERLAPPEDWINDOW, CW_USEDEFAULT, CW_USEDEFAULT, 600, 800, NULL, NULL, hInstance, NULL);
if (hwnd == NULL) {
return 1;
}
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
```
阅读全文
相关推荐
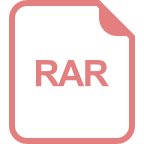
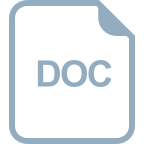
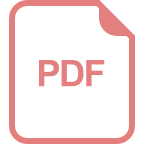
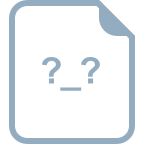
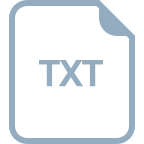
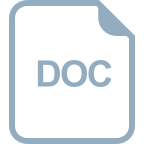
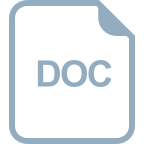







