自动发射子弹c语言,C语言代码实现飞机大战
时间: 2023-09-28 16:13:28 浏览: 74
以下是一个简单的 C 语言代码实现飞机大战中自动发射子弹的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 80
#define HEIGHT 25
typedef struct {
int x, y;
} Point;
void gotoxy(int x, int y) {
COORD coord = { x, y };
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), coord);
}
void drawChar(int x, int y, char c) {
gotoxy(x, y);
putchar(c);
}
void drawString(int x, int y, char* str) {
gotoxy(x, y);
printf("%s", str);
}
void drawLine(int x1, int y1, int x2, int y2, char c) {
int dx = x2 - x1, dy = y2 - y1;
int steps = abs(dx) > abs(dy) ? abs(dx) : abs(dy);
float xinc = dx / (float)steps;
float yinc = dy / (float)steps;
float x = x1, y = y1;
int i;
for (i = 0; i < steps; i++) {
drawChar((int)x, (int)y, c);
x += xinc;
y += yinc;
}
}
void clearScreen() {
system("cls");
}
void drawPlane(int x, int y) {
drawChar(x, y, 'A');
drawChar(x - 1, y + 1, '<');
drawChar(x + 1, y + 1, '>');
drawChar(x, y + 2, '|');
}
void drawBullet(int x, int y) {
drawChar(x, y, '|');
}
void moveBullet(Point* bullet) {
bullet->y--;
}
int main() {
int planeX = WIDTH / 2;
int planeY = HEIGHT - 4;
int bulletX = planeX, bulletY = planeY - 1;
int score = 0;
srand((unsigned int)time(NULL));
while (1) {
clearScreen();
drawPlane(planeX, planeY);
drawBullet(bulletX, bulletY);
drawString(0, 0, "Score: ");
printf("%d", score);
if (bulletY == 0) {
bulletX = planeX;
bulletY = planeY - 1;
}
else {
moveBullet(&bulletX, &bulletY);
}
if (_kbhit()) {
char c = _getch();
if (c == 'a' && planeX > 0) {
planeX--;
}
else if (c == 'd' && planeX < WIDTH - 1) {
planeX++;
}
else if (c == ' ') {
bulletX = planeX;
bulletY = planeY - 1;
}
}
Sleep(50);
}
return 0;
}
```
该示例代码使用了 Windows 系统下的一些 API 和库函数,运行时需要在 Windows 系统下进行编译和运行。
相关推荐
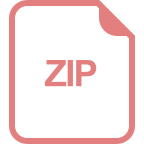
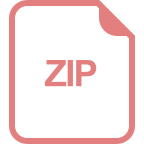














