matlab中双电梯仿真代码
时间: 2023-08-07 07:02:26 浏览: 61
以下是一个简单的 MATLAB 双电梯仿真代码,你可以根据自己的需要进行修改和扩展:
```matlab
%% 双电梯仿真代码
% 参数设定
floors = 10; % 楼层数
people = 20; % 乘客数
elevator_capacity = 8; % 电梯容量
steps_per_floor = 15; % 每层楼所需时间
elevator_speed = 3; % 电梯速度
elevator_delay = 2; % 电梯延迟时间
% 初始化电梯状态
elevator1 = struct('position', 1, 'direction', 1, 'queue', [], 'timer', 0, 'occupants', []);
elevator2 = struct('position', 1, 'direction', 1, 'queue', [], 'timer', 0, 'occupants', []);
% 初始化乘客状态
people_waiting = struct('floor', [], 'destination', [], 'timer', []);
% 初始化计时器
time = 0;
% 生成随机乘客
for i = 1:people
people_waiting(i).floor = randi(floors);
people_waiting(i).destination = randi(floors);
while people_waiting(i).destination == people_waiting(i).floor
people_waiting(i).destination = randi(floors);
end
end
% 主循环
while true
% 更新计时器
time = time + 1;
% 处理电梯内的乘客
if ~isempty(elevator1.occupants)
for i = 1:length(elevator1.occupants)
elevator1.occupants(i).timer = elevator1.occupants(i).timer - 1;
if elevator1.occupants(i).timer <= 0
elevator1.occupants(i) = [];
end
end
end
if ~isempty(elevator2.occupants)
for i = 1:length(elevator2.occupants)
elevator2.occupants(i).timer = elevator2.occupants(i).timer - 1;
if elevator2.occupants(i).timer <= 0
elevator2.occupants(i) = [];
end
end
end
% 处理电梯内的任务队列
if ~isempty(elevator1.queue)
if elevator1.timer > 0
elevator1.timer = elevator1.timer - 1;
else
elevator1.position = elevator1.queue(1);
elevator1.queue(1) = [];
elevator1.timer = elevator_delay;
end
end
if ~isempty(elevator2.queue)
if elevator2.timer > 0
elevator2.timer = elevator2.timer - 1;
else
elevator2.position = elevator2.queue(1);
elevator2.queue(1) = [];
elevator2.timer = elevator_delay;
end
end
% 处理等待电梯的乘客
for i = 1:length(people_waiting)
if people_waiting(i).timer > 0
people_waiting(i).timer = people_waiting(i).timer - 1;
else
if isempty(elevator1.queue) && isempty(elevator2.queue)
% 如果两台电梯都没有任务,则随机选择一台
if randi(2) == 1
elevator1.queue = [elevator1.queue, people_waiting(i).floor];
else
elevator2.queue = [elevator2.queue, people_waiting(i).floor];
end
elseif isempty(elevator1.queue)
% 如果电梯1没有任务,则选择电梯1
elevator1.queue = [elevator1.queue, people_waiting(i).floor];
elseif isempty(elevator2.queue)
% 如果电梯2没有任务,则选择电梯2
elevator2.queue = [elevator2.queue, people_waiting(i).floor];
else
% 如果两台电梯都有任务,则选择距离更近的电梯
if abs(elevator1.position - people_waiting(i).floor) <= abs(elevator2.position - people_waiting(i).floor)
elevator1.queue = [elevator1.queue, people_waiting(i).floor];
else
elevator2.queue = [elevator2.queue, people_waiting(i).floor];
end
end
people_waiting(i).timer = steps_per_floor;
end
end
% 处理电梯的运行状态
if isempty(elevator1.queue) && isempty(elevator2.queue)
% 如果两台电梯都没有任务,则不动
elseif isempty(elevator1.queue)
% 如果电梯1没有任务,则电梯2运行
if elevator2.direction == 1
if elevator2.position < max(elevator2.queue)
elevator2.position = elevator2.position + elevator_speed;
else
elevator2.direction = -1;
end
else
if elevator2.position > min(elevator2.queue)
elevator2.position = elevator2.position - elevator_speed;
else
elevator2.direction = 1;
end
end
elseif isempty(elevator2.queue)
% 如果电梯2没有任务,则电梯1运行
if elevator1.direction == 1
if elevator1.position < max(elevator1.queue)
elevator1.position = elevator1.position + elevator_speed;
else
elevator1.direction = -1;
end
else
if elevator1.position > min(elevator1.queue)
elevator1.position = elevator1.position - elevator_speed;
else
elevator1.direction = 1;
end
end
else
% 如果两台电梯都有任务,则按距离更近的电梯运行
if abs(elevator1.position - elevator1.queue(1)) <= abs(elevator2.position - elevator2.queue(1))
if elevator1.direction == 1
if elevator1.position < max(elevator1.queue)
elevator1.position = elevator1.position + elevator_speed;
else
elevator1.direction = -1;
end
else
if elevator1.position > min(elevator1.queue)
elevator1.position = elevator1.position - elevator_speed;
else
elevator1.direction = 1;
end
end
else
if elevator2.direction == 1
if elevator2.position < max(elevator2.queue)
elevator2.position = elevator2.position + elevator_speed;
else
elevator2.direction = -1;
end
else
if elevator2.position > min(elevator2.queue)
elevator2.position = elevator2.position - elevator_speed;
else
elevator2.direction = 1;
end
end
end
end
% 处理电梯内的乘客任务
if ~isempty(elevator1.occupants)
for i = 1:length(elevator1.occupants)
if elevator1.occupants(i).destination == elevator1.position
elevator1.occupants(i).timer = steps_per_floor;
elevator1.occupants(i).destination = [];
end
end
elevator1.occupants = elevator1.occupants(~arrayfun(@(x) isempty(x.destination), elevator1.occupants));
end
if ~isempty(elevator2.occupants)
for i = 1:length(elevator2.occupants)
if elevator2.occupants(i).destination == elevator2.position
elevator2.occupants(i).timer = steps_per_floor;
elevator2.occupants(i).destination = [];
end
end
elevator2.occupants = elevator2.occupants(~arrayfun(@(x) isempty(x.destination), elevator2.occupants));
end
% 判断是否完成所有任务
if isempty(elevator1.queue) && isempty(elevator2.queue) && isempty(people_waiting) && isempty(elevator1.occupants) && isempty(elevator2.occupants)
break;
end
end
% 输出结果
disp(['总用时:', num2str(time), 's']);
```
注意,这只是一个简单的双电梯仿真代码,还有很多需要完善的地方,例如电梯的调度算法、乘客的等待策略、电梯内部的行为等。如果需要更加精细的模拟,请根据实际需要进行修改和扩展。
相关推荐
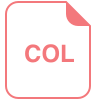
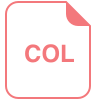
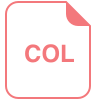
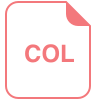
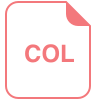










