python计算两个表格中的数据的spearman秩相关系数
时间: 2024-05-14 16:15:35 浏览: 187
要计算两个表格的Spearman秩相关系数,可以使用Python中的SciPy库。以下是一个示例代码:
```python
import pandas as pd
from scipy.stats import spearmanr
# 读取两个表格数据
df1 = pd.read_csv('table1.csv')
df2 = pd.read_csv('table2.csv')
# 提取需要计算相关系数的列
col1 = df1['column1']
col2 = df2['column2']
# 计算Spearman秩相关系数
corr, _ = spearmanr(col1, col2)
print('Spearman秩相关系数:', corr)
```
其中,`table1.csv`和`table2.csv`分别是两个表格的数据文件,`column1`和`column2`分别是需要计算相关系数的列名。最后输出的`corr`即为Spearman秩相关系数的值。
相关问题
python计算两个表格中的数据的spearman秩相关系数,并将结果生成一个新的表格
好的,需要使用Python中的pandas和scipy库。以下是代码示例:
```python
import pandas as pd
from scipy.stats import spearmanr
# 读取两个表格
table1 = pd.read_csv('table1.csv')
table2 = pd.read_csv('table2.csv')
# 计算Spearman秩相关系数
corr, p_value = spearmanr(table1['column1'], table2['column2'])
# 创建新的表格
result_table = pd.DataFrame({'Spearman Correlation': [corr], 'p-value': [p_value]})
# 将结果保存到CSV文件中
result_table.to_csv('result_table.csv', index=False)
```
请注意,需要将`column1`和`column2`替换为要比较的两个表格中的列的名称,以及将文件名替换为实际使用的文件名。
python numpy 计算多列数据的spearman相关系数矩阵
在Python中,可以使用NumPy库配合SciPy库来计算多列数据的Spearman秩相关系数矩阵。Spearman秩相关系数是一种非参数统计方法,它衡量的是两个变量之间的相对位置关系,而不是具体的数值大小。
首先,你需要安装这两个库,如果还没有安装,可以使用pip命令:
```bash
pip install numpy scipy
```
然后,你可以按照以下步骤计算多列数据的Spearman相关系数矩阵:
```python
import numpy as np
from scipy.stats import spearmanr
# 假设你有这样一个二维数组,包含多列数据
data = np.array([[data1], [data2], ..., [dataN]]) # data1, data2, ... 是每一列的数据
# 将数据转换为秩
ranked_data = np.apply_along_axis(rank, axis=0, arr=data)
# 计算Spearman相关系数矩阵
correlation_matrix = np.corrcoef(ranked_data.T)
# 对角线赋值为1(因为每个变量与自身的秩相关系数为1)
np.fill_diagonal(correlation_matrix, 1)
print("Spearman相关系数矩阵:")
print(correlation_matrix)
```
在这个例子中,`rank()`函数用于将每列数据转换为秩,`corrcoef()`函数则计算它们的协方差矩阵,最后填充对角线使得自相关系数为1。
阅读全文
相关推荐
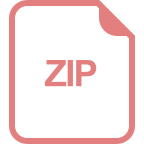
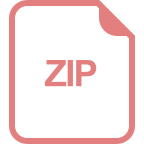
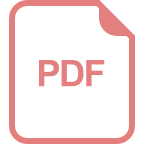













