public int compareTo(Good good) { return this.price.compareTo(good.price); }
时间: 2024-04-19 08:25:31 浏览: 19
这是一个实现了 Comparable 接口的 compareTo 方法。在这段代码中,假设你有一个名为 Good 的类,它具有一个 price 属性,并且你希望能够根据 price 属性对 Good 对象进行比较。
compareTo 方法是 Comparable 接口中定义的方法,它用于比较当前对象与传入的另一个 Good 对象。在这里,使用了 this.price.compareTo(good.price) 来比较两个 Good 对象的 price 属性。
this.price 是指当前对象的 price 属性,good.price 是指传入的另一个 Good 对象的 price 属性。调用 compareTo 方法后,会返回一个整数值,表示两个对象的比较结果。
具体来说,当返回值为负数时,表示当前对象的 price 属性小于传入对象的 price 属性;返回值为零时,表示两个对象的 price 属性相等;返回值为正数时,表示当前对象的 price 属性大于传入对象的 price 属性。
通过实现 Comparable 接口和 compareTo 方法,你可以在需要对 Good 对象进行排序或比较操作时使用标准的排序方法(如排序算法或集合类的排序方法)。
相关问题
public int compareTo(Good good) { return this.price.compareTo(good.price);
这是一个在 Java 中实现比较方法 `compareTo()` 的示例代码。在这里,假设 `Good` 类具有一个 `price` 字段,它实现了 `Comparable` 接口。
`compareTo()` 方法用于比较调用对象和传入的 `good` 对象的价格。`this.price.compareTo(good.price)` 表示将调用对象的价格与传入对象的价格进行比较。`compareTo()` 方法返回一个整数值,具体含义如下:
- 如果调用对象的价格小于传入对象的价格,返回一个负整数。
- 如果调用对象的价格等于传入对象的价格,返回 0。
- 如果调用对象的价格大于传入对象的价格,返回一个正整数。
通过使用 `compareTo()` 方法,你可以对 `Good` 对象进行比较并按照价格进行排序。请注意,在实现 `compareTo()` 方法时,你需要确保 `price` 字段是可比较的,例如是一个实现了 `Comparable` 接口的类型,如 `Integer` 或 `Double`。
解释一下这行代码public int CompareTo(Book other)
### 回答1:
这行代码是用来实现比较两个Book对象大小的方法。其中,CompareTo是接口IComparable中定义的方法,其返回值为int类型,表示两个对象的大小关系。这个方法接收一个Book对象作为参数,和当前Book对象进行比较,如果当前对象小于参数对象,则返回一个负数,如果当前对象等于参数对象,则返回0,如果当前对象大于参数对象,则返回一个正数。通过这个方法,可以方便地对Book对象进行排序等操作。
### 回答2:
这行代码 `public int CompareTo(Book other)` 是一个比较方法,用于比较一个 `Book` 对象与另一个 `Book` 对象 `other` 的大小关系,并返回一个整数值。
在这个比较方法中,我们使用了泛型类型参数 `Book`,它表明这个方法是定义在 `Book` 类中的一个实例方法。`public` 关键字表示这个方法是公共的,可以在其他类中访问和使用。
`CompareTo` 方法的返回类型是 `int`,也就是整数类型。它的返回值有以下几种可能性:
- 如果当前的 `Book` 对象小于 `other`,则返回一个负整数。
- 如果当前的 `Book` 对象等于 `other`,则返回0。
- 如果当前的 `Book` 对象大于 `other`,则返回一个正整数。
通过返回不同的整数值,我们可以对任意两个 `Book` 对象进行大小比较。
这个 `CompareTo` 方法的具体实现可能依赖于 `Book` 类的内部实现和属性。一般而言,我们可以基于 `Book` 对象的某个属性(如书名、作者、出版日期等)进行比较,并返回相应的结果。
例如,假设 `Book` 类有一个属性 `title` 表示书名,我们可以在 `CompareTo` 方法中比较两个 `Book` 对象的 `title` 属性,并返回它们之间的字典顺序关系,或者根据字母的 ASCII 码进行比较。具体的实现方式根据需求和场景的不同而有所差异。
### 回答3:
这行代码`public int CompareTo(Book other)`是一个方法的声明语句,其中`CompareTo`是该方法的名称,`public`表示该方法是公有的,`int`表示该方法的返回类型是整数类型。
这个方法是用来比较两个`Book`对象之间的大小关系,并返回一个整数值。它需要传入一个`Book`类型的参数`other`,表示要进行比较的另一个书籍对象。
在这个方法的实现中,我们可以自定义比较规则。假设我们要根据书籍的价格进行比较,可以通过比较两个书籍对象的价格属性来确定它们的大小关系。如果当前书籍的价格小于参数书籍的价格,则返回一个负数;如果当前书籍的价格大于参数书籍的价格,则返回一个正数;如果两个书籍的价格相同,则返回0。
例如,我们可以实现如下的比较逻辑:
```java
public int CompareTo(Book other) {
return this.price - other.price;
}
```
在上述代码中,我们假设`Book`类中有一个`price`属性来表示书籍的价格。通过对当前书籍对象的价格减去参数书籍的价格,可以得到两者的价格差。根据差值的正负来确定它们的大小关系,从而返回相应的整数值。
这个`CompareTo`方法可以用于排序算法中,比如在集合排序、二叉搜索树、堆等数据结构中,根据书籍的价格属性对书籍对象进行排序。此外,它还可以作为比较器传入`Arrays.sort()`或`Collections.sort()`等方法中,用于进行书籍对象的排序操作。
相关推荐
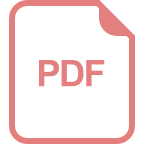
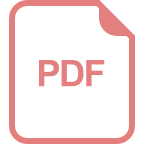











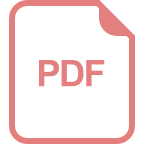
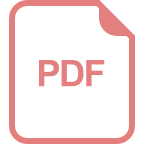