编写手机类(MobilePhone),含有type(型号,String类型)、price(价格,int类型)属性,要求该类实现Comparable接口,重写compareTo方法,实现按照price的大小来确定两个手机对象的大小关系。 在链表中添加三个手机对象(从键盘输入),通过Collections类的sort方法对链表中的对象按照price升序排序。输入第四个手机对象的信息,并查找它的price是否与链表中某个对象的price相同。
时间: 2024-03-07 07:48:41 浏览: 56
好的,我可以为您编写这个MobilePhone类和实现类。
MobilePhone类:
```java
public class MobilePhone implements Comparable<MobilePhone>{
private String type;
private int price;
public MobilePhone(String type, int price) {
this.type = type;
this.price = price;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
@Override
public int compareTo(MobilePhone other) {
return this.price - other.getPrice();
}
}
```
实现类:
```java
import java.util.*;
public class MobilePhoneTest {
public static void main(String[] args) {
List<MobilePhone> phoneList = new LinkedList<>();
Scanner scanner = new Scanner(System.in);
for(int i = 0; i < 3; i++) {
System.out.println("请输入手机型号:");
String type = scanner.nextLine();
System.out.println("请输入手机价格:");
int price = scanner.nextInt();
scanner.nextLine(); // 消耗掉输入的回车符
MobilePhone phone = new MobilePhone(type, price);
phoneList.add(phone);
}
Collections.sort(phoneList);
for(MobilePhone phone : phoneList) {
System.out.println("手机型号:" + phone.getType() + ",价格:" + phone.getPrice());
}
System.out.println("请输入第四个手机型号:");
String type = scanner.nextLine();
System.out.println("请输入第四个手机价格:");
int price = scanner.nextInt();
boolean found = false;
for(MobilePhone phone : phoneList) {
if(phone.getPrice() == price) {
found = true;
System.out.println("第四个手机价格与链表中手机价格相同");
break;
}
}
if(!found) {
System.out.println("第四个手机价格与链表中手机价格不同");
}
}
}
```
希望这个例子可以帮助您理解如何实现一个实现了Comparable接口的MobilePhone类,以及如何使用Collections类对链表中的MobilePhone对象按照price升序排序,并查找特定的MobilePhone对象的price是否与链表中某个对象的price相同。
相关推荐
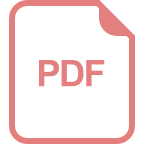
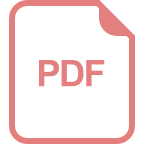







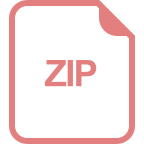
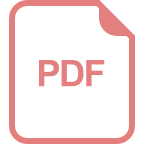
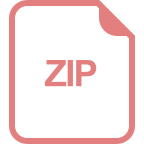