python contours,_ =cv2.findContours
时间: 2024-08-13 09:00:50 浏览: 51
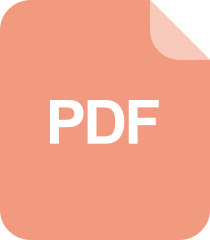
python+opencv实现移动侦测(帧差法)
在OpenCV (Computer Vision Library) 中,`cv2.findContours()` 函数是用于从二进制图像中查找轮廓(contours)。函数返回两个值,第一个是找到的轮廓列表(如果有多于一个轮廓,可能是一个二维数组),第二个值通常是`None`,因为在旧版本的OpenCV中它会被忽略。
调用这个函数的基本语法通常是这样的:
```python
contours, hierarchy = cv2.findContours(image, mode, method)
```
其中,
- `image` 是输入的灰度或二值图像。
- `mode` 是指定寻找轮廓的方式,例如 `cv2.RETR_EXTERNAL` 表示只查找外部轮廓,`cv2.RETR_LIST` 则获取所有轮廓。
- `method` 是边缘检测方法,如 `cv2.CHAIN_APPROX_SIMPLE` 或 `cv2.CHAIN_APPROX_NONE` 等。
找到的轮廓可以用在后续的各种图像处理任务中,如形状分析、物体识别等。
阅读全文
相关推荐
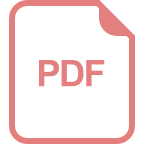
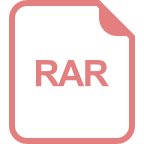















