unordered_set find
时间: 2023-11-24 17:46:26 浏览: 84
unordered_set的find函数用于在unordered_set容器中查找特定元素。它返回一个迭代器,指向查找到的元素,如果未找到,则返回指向unordered_set容器末尾的迭代器。
以下是使用unordered_set的find函数的示例代码:
```cpp
#include <iostream>
#include <unordered_set>
int main() {
std::unordered_set<int> mySet = {1, 2, 3, 4, 5};
auto it = mySet.find(3);
if (it != mySet.end()) {
std::cout << "Element found: " << *it << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
return 0;
}
```
输出结果:
```
Element found: 3
```
如果要检查unordered_set容器中是否存在某个元素,可以使用find函数来查找元素。如果返回的迭代器指向容器末尾,表示元素未找到。
相关问题
unordered_set find()函数
unordered_set 的 find() 函数用于在无序集合中查找特定元素,并返回指向该元素的迭代器。如果该元素不存在于集合中,则返回指向 unordered_set 结尾的迭代器(即 unordered_set::end())。
find() 函数的时间复杂度是 O(1),因为它使用哈希表来实现快速查找。因此,unordered_set 是一种非常高效的数据结构,可以用于需要快速查找和去重的场合。
unordered_set中find
`unordered_set` 是 C++ STL 中的一个容器,它是一个哈希表,可以用于存储一组不重复的元素。`unordered_set::find` 函数用于在 `unordered_set` 容器中查找指定的元素。
`unordered_set::find` 函数接受一个参数,即要查找的元素。如果该元素在容器中存在,则返回一个迭代器,指向该元素所在的位置;如果该元素不存在,则返回一个指向容器末尾的迭代器。
下面是一个示例代码:
```c++
#include <iostream>
#include <unordered_set>
int main() {
std::unordered_set<int> myset = {1, 2, 3, 4, 5};
// 查找元素 3
auto it = myset.find(3);
if (it != myset.end()) {
std::cout << "Found element: " << *it << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
// 查找元素 6
it = myset.find(6);
if (it != myset.end()) {
std::cout << "Found element: " << *it << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
return 0;
}
```
输出结果为:
```
Found element: 3
Element not found
```
在上述代码中,我们首先创建了一个 `unordered_set` 容器,并向其中插入了一些元素。然后,我们使用 `find` 函数查找容器中的元素。在第一次查找中,元素 3 存在于容器中,因此 `find` 函数返回了指向元素 3 的迭代器,输出了 `Found element: 3`;而在第二次查找中,元素 6 不存在于容器中,因此 `find` 函数返回了指向容器末尾的迭代器,输出了 `Element not found`。
阅读全文
相关推荐
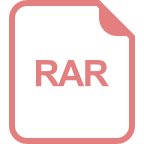
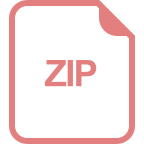
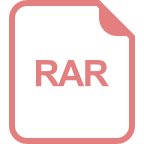












