做个使用vue做一个发表评论的页面
时间: 2024-11-13 22:24:59 浏览: 0
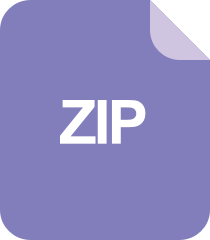
使用SpringBoot + Vue3 + TypeScript做的一个博客系统源码.zip
在Vue.js中创建一个发表评论的页面通常涉及以下几个步骤:
1. **组件设计**:
- 定义一个`CommentForm`组件,用于用户输入评论内容。这个组件通常包括输入框(textarea)和提交按钮。
```html
<template>
<div class="comment-form">
<input type="text" v-model="newComment" placeholder="请输入评论内容...">
<button @click.prevent="submitComment">发布</button>
</div>
</template>
<script>
export default {
data() {
return {
newComment: ''
};
},
methods: {
submitComment() {
// 发送评论到服务器的逻辑
}
}
};
</script>
```
2. **表单验证**:
可能需要对用户输入进行一些基本的验证,比如检查是否为空等。
3. **与服务器交互**:
在`submitComment`方法中,你需要连接到后端API,通常是通过axios这样的库发送HTTP请求。示例代码如下:
```js
methods: {
async submitComment() {
if (this.newComment.trim()) {
try {
await axios.post('/api/comments', { content: this.newComment });
this.newComment = '';
// 显示成功提示或清空评论字段
} catch (error) {
console.error('Error submitting comment:', error);
}
}
}
}
```
4. **模板嵌套或布局**:
如果有多个评论已经存在,你可以显示在一个列表里,每个评论作为一个子组件,如`CommentItem.vue`。在父组件中遍历评论数据渲染。
```html
<template>
<div>
<CommentForm @submit="addComment"></CommentForm>
<comment-item v-for="(comment, index) in comments" :key="index" :comment="comment" />
</div>
</template>
```
5. **状态管理**:
如果应用较大,可以考虑使用Vuex来管理全局状态,如评论数组。
阅读全文
相关推荐
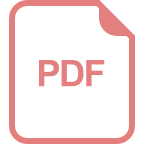
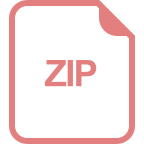
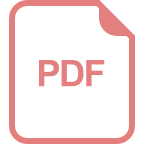
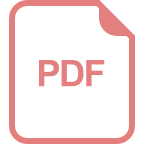
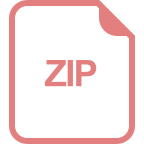
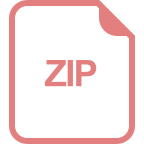
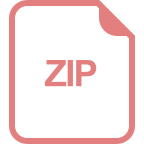
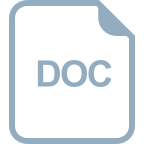
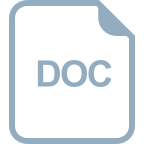
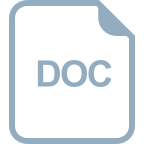
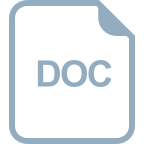






