springboot项目数据导出excel表
时间: 2023-12-18 16:03:28 浏览: 11
可以使用POI库来操作Excel文件,以下是实现数据导出Excel表格的步骤:
1. 添加POI库的依赖:在pom.xml文件中添加以下依赖
```
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
```
2. 创建Excel文件:使用POI创建一个工作簿对象,然后创建一个工作表对象
```
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
```
3. 添加表头:在工作表中添加表头,可以使用POI的Row和Cell对象
```
Row row = sheet.createRow(0);
Cell cell1 = row.createCell(0);
cell1.setCellValue("列1");
Cell cell2 = row.createCell(1);
cell2.setCellValue("列2");
...
```
4. 添加数据:将数据添加到工作表中,可以使用POI的Row和Cell对象
```
for(int i = 0; i < dataList.size(); i++) {
Row row = sheet.createRow(i + 1);
Cell cell1 = row.createCell(0);
cell1.setCellValue(dataList.get(i).getField1());
Cell cell2 = row.createCell(1);
cell2.setCellValue(dataList.get(i).getField2());
...
}
```
5. 输出Excel文件:将工作簿对象输出到Excel文件中
```
FileOutputStream fileOut = new FileOutputStream("data.xlsx");
workbook.write(fileOut);
fileOut.close();
workbook.close();
```
完整代码示例:
```
@GetMapping("/export")
public void exportData(HttpServletResponse response) throws Exception {
// 查询数据
List<Data> dataList = dataService.getDataList();
// 创建Excel文件
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
// 添加表头
Row row = sheet.createRow(0);
Cell cell1 = row.createCell(0);
cell1.setCellValue("列1");
Cell cell2 = row.createCell(1);
cell2.setCellValue("列2");
...
// 添加数据
for(int i = 0; i < dataList.size(); i++) {
Row row = sheet.createRow(i + 1);
Cell cell1 = row.createCell(0);
cell1.setCellValue(dataList.get(i).getField1());
Cell cell2 = row.createCell(1);
cell2.setCellValue(dataList.get(i).getField2());
...
}
// 输出Excel文件
response.setContentType("application/vnd.ms-excel");
response.setHeader("Content-Disposition", "attachment; filename=data.xlsx");
OutputStream outputStream = response.getOutputStream();
workbook.write(outputStream);
outputStream.flush();
outputStream.close();
workbook.close();
}
```
相关推荐
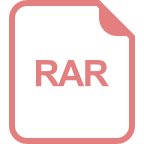
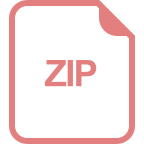
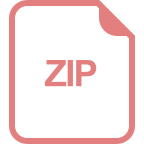














