给我一段用dfs生成最短路径的代码
时间: 2024-10-20 21:13:17 浏览: 20
DFS(深度优先搜索)通常用于查找图中的连通分量、是否存在环等问题,而Dijkstra算法或A*算法更适合找到最短路径。不过,如果你想了解如何利用DFS探索所有可能的路径并找到其中最短的一个(假设每个边的权重都是正数),可以使用启发式搜索结合记忆化技术。下面是一个简单的Python示例,展示如何用DFS找出两个节点之间的最短路径,但注意这并非标准的最短路径求解方法:
```python
from collections import defaultdict
def shortest_path_with_dfs(graph, start, end):
distances = {node: float('inf') for node in graph}
distances[start] = 0
path = {}
def dfs(node, current_path, curr_distance):
if node == end:
# 如果到达目标节点,更新最短距离
if curr_distance < distances[node]:
distances[node] = curr_distance
path[end] = current_path + [end]
else:
for neighbor, weight in graph[node].items():
new_distance = curr_distance + weight
if new_distance < distances[neighbor]:
distances[neighbor] = new_distance
path[neighbor] = current_path + [neighbor]
dfs(neighbor, path[neighbor], new_distance)
dfs(start, [], 0)
return distances[end], path[end] if end in path else None
# 使用示例,这里假设graph是一个邻接权重字典
graph = {
'A': {'B': 1, 'C': 4},
'B': {'A': 1, 'C': 2, 'D': 5},
'C': {'A': 4, 'B': 2, 'D': 1},
'D': {'B': 5, 'C': 1}
}
shortest, path = shortest_path_with_dfs(graph, 'A', 'D')
print(f"Shortest distance from A to D is: {shortest}")
print(f"Path: {path}")
```
阅读全文
相关推荐
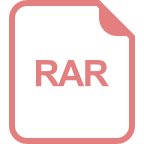
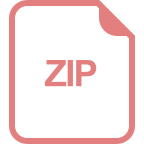
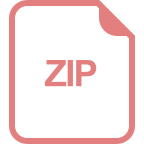



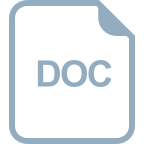
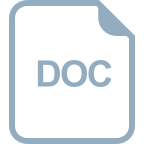
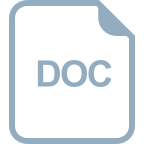
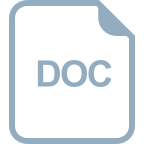
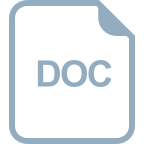
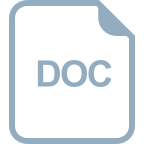
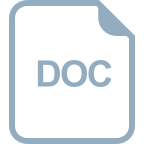
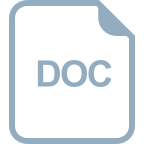
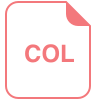
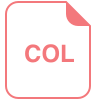
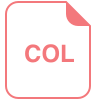
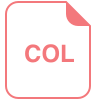
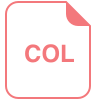