java练习图书借阅管理系统
时间: 2023-10-17 09:14:04 浏览: 41
好的,我可以为您提供一个简单的Java练习图书借阅管理系统的实现思路。以下是实现的基本步骤:
1. 创建图书类 Book,包含属性:书名、作者、出版社、ISBN 等信息。
2. 创建用户类 User,包含属性:用户名、密码、已借图书列表等信息。
3. 创建图书馆类 Library,包含属性:图书列表、用户列表等信息,以及方法:添加图书、删除图书、借出图书、归还图书等。
4. 创建主程序类 Main,实现用户登录和菜单选择等功能。
具体的代码实现可以参考以下示例:
Book.java:
```java
public class Book {
private String title;
private String author;
private String publisher;
private String ISBN;
public Book(String title, String author, String publisher, String ISBN) {
this.title = title;
this.author = author;
this.publisher = publisher;
this.ISBN = ISBN;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getPublisher() {
return publisher;
}
public String getISBN() {
return ISBN;
}
@Override
public String toString() {
return "Book{" +
"title='" + title + '\'' +
", author='" + author + '\'' +
", publisher='" + publisher + '\'' +
", ISBN='" + ISBN + '\'' +
'}';
}
}
```
User.java:
```java
import java.util.ArrayList;
import java.util.List;
public class User {
private String username;
private String password;
private List<Book> borrowedBooks;
public User(String username, String password) {
this.username = username;
this.password = password;
this.borrowedBooks = new ArrayList<>();
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
public boolean borrowBook(Book book) {
if (borrowedBooks.size() >= 3) {
System.out.println("You have borrowed too many books!");
return false;
} else {
borrowedBooks.add(book);
return true;
}
}
public boolean returnBook(Book book) {
if (borrowedBooks.contains(book)) {
borrowedBooks.remove(book);
return true;
} else {
System.out.println("You haven't borrowed this book!");
return false;
}
}
public List<Book> getBorrowedBooks() {
return borrowedBooks;
}
}
```
Library.java:
```java
import java.util.ArrayList;
import java.util.List;
public class Library {
private List<Book> books;
private List<User> users;
public Library() {
this.books = new ArrayList<>();
this.users = new ArrayList<>();
}
public void addBook(Book book) {
books.add(book);
}
public void removeBook(Book book) {
books.remove(book);
}
public void addUser(User user) {
users.add(user);
}
public void removeUser(User user) {
users.remove(user);
}
public boolean borrowBook(User user, Book book) {
if (!books.contains(book)) {
System.out.println("The book is not available!");
return false;
} else {
return user.borrowBook(book);
}
}
public void returnBook(User user, Book book) {
if (user.returnBook(book)) {
books.add(book);
}
}
public List<Book> getBooks() {
return books;
}
public List<User> getUsers() {
return users;
}
}
```
Main.java:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Library library = new Library();
Book book1 = new Book("Java Programming", "John Smith", "Pearson", "978-0134685991");
Book book2 = new Book("Data Structures and Algorithms", "Robert Lafore", "SAMS Publishing", "978-0672324536");
Book book3 = new Book("Clean Code", "Robert C. Martin", "Prentice Hall", "978-0132350884");
library.addBook(book1);
library.addBook(book2);
library.addBook(book3);
User user1 = new User("alice", "123456");
User user2 = new User("bob", "password");
library.addUser(user1);
library.addUser(user2);
Scanner scanner = new Scanner(System.in);
System.out.println("Welcome to the Library Management System!");
System.out.print("Enter your username: ");
String username = scanner.nextLine();
System.out.print("Enter your password: ");
String password = scanner.nextLine();
User currentUser = null;
for (User user : library.getUsers()) {
if (user.getUsername().equals(username) && user.getPassword().equals(password)) {
currentUser = user;
break;
}
}
if (currentUser == null) {
System.out.println("Invalid username or password!");
return;
}
while (true) {
System.out.println("Please select an option:");
System.out.println("1. View available books");
System.out.println("2. Borrow a book");
System.out.println("3. Return a book");
System.out.println("4. View borrowed books");
System.out.println("5. Exit");
int choice = scanner.nextInt();
scanner.nextLine(); // consume the newline character
switch (choice) {
case 1:
System.out.println("Available books:");
for (Book book : library.getBooks()) {
System.out.println(book);
}
break;
case 2:
System.out.print("Enter the ISBN of the book you want to borrow: ");
String ISBN = scanner.nextLine();
Book bookToBorrow = null;
for (Book book : library.getBooks()) {
if (book.getISBN().equals(ISBN)) {
bookToBorrow = book;
break;
}
}
if (bookToBorrow == null) {
System.out.println("Invalid ISBN!");
} else {
if (library.borrowBook(currentUser, bookToBorrow)) {
System.out.println("You have successfully borrowed the book!");
}
}
break;
case 3:
System.out.print("Enter the ISBN of the book you want to return: ");
ISBN = scanner.nextLine();
Book bookToReturn = null;
for (Book book : currentUser.getBorrowedBooks()) {
if (book.getISBN().equals(ISBN)) {
bookToReturn = book;
break;
}
}
if (bookToReturn == null) {
System.out.println("Invalid ISBN!");
} else {
library.returnBook(currentUser, bookToReturn);
System.out.println("You have successfully returned the book!");
}
break;
case 4:
System.out.println("Borrowed books:");
for (Book book : currentUser.getBorrowedBooks()) {
System.out.println(book);
}
break;
case 5:
System.out.println("Goodbye!");
return;
default:
System.out.println("Invalid choice!");
break;
}
}
}
}
```
这个图书借阅管理系统实现了基本的借书、还书、查看图书等功能,您可以在此基础上继续扩展和优化。
相关推荐
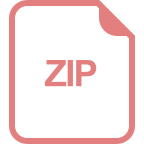
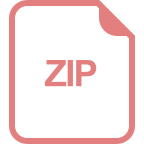
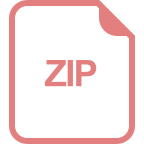














