Python生成一个跳动的心的代码
时间: 2024-10-05 15:03:24 浏览: 18
在Python中,我们可以使用ASCII艺术或者更现代的库如`pygame`来创建动态效果,但这里我们展示一个简单的ASCII艺术实现"跳动的心"。由于文本环境的限制,我们将创建一个静态的"心形图案",然后每隔一段时间打印一次,模拟"跳动"的感觉。
```python
import time
def print_heart():
for i in range(4):
print(" " * (i*5) + "*" * (8-i*2) + " "*((4-i)*5) + "****" + " "*((4-i)*5) + "*" * (8-i*2) + " " * (i*5))
print("静止状态的心")
time.sleep(1)
print_heart()
print("\n第一次跳动")
time.sleep(1)
print_heart()
# 更多的循环可以增加"跳动"的效果
# 这里仅演示了两次,你可以根据需要修改循环次数
```
这个代码会先显示一个静态的心形图案,然后每过一秒"跳动"一次。如果你想让心形真的"跳动"起来,那么最好使用图形界面库如pygame。
相关问题
生成一个跳动的心的代码
这是一段使用Python Turtle库绘制一个跳动的心的代码:
```python
import turtle
t = turtle.Turtle()
# 设置画笔颜色和大小
t.color('red', 'pink')
t.pensize(3)
# 绘制心形
t.begin_fill()
t.left(45)
t.forward(150)
t.circle(75, 180)
t.right(90)
t.circle(75, 180)
t.forward(150)
t.end_fill()
# 移动画笔到下一个位置
t.penup()
t.goto(0, -50)
t.pendown()
# 绘制跳动的心
for i in range(1, 6):
t.begin_fill()
t.circle(25*i, 180)
t.circle(-25*i, 180)
t.end_fill()
# 隐藏画笔
t.hideturtle()
turtle.done()
```
运行代码后,会弹出一个窗口,显示跳动的心的动画效果。可以使用Turtle库的其他函数和方法,调整心的大小、颜色、跳动的速度等。
python爱心跳动游戏代码
以下是一个简单的Python爱心跳动游戏代码,使用了Tkinter库和数学函数生成器来实现:
```python
import random
from math import sin, cos, pi, log
from tkinter import *
# 设置画布大小
WIDTH = 800
HEIGHT = 600
# 设置心的颜色
HEART_COLOR = "#ff8181"
# 设置放大比例
IMAGE_ENLARGE = 20
# 定义心的函数生成器
def heart_function(t, shrink_ratio: float = IMAGE_ENLARGE):
"""
“爱心函数生成器”
:param shrink_ratio: 放大比例
:param t: 参数
:return: 坐标
"""
# 基础函数
x = 16 * (sin(t) ** 3)
y = -(13 * cos(t) - 5 * cos(2 * t) - 2 * cos(3 * t) - cos(4 * t))
# 放大
x *= shrink_ratio
y *= shrink_ratio
# 移到画布中央
x += WIDTH / 2
y += HEIGHT / 2
return x, y
# 创建画布
root = Tk()
root.title("爱心跳动游戏")
canvas = Canvas(root, width=WIDTH, height=HEIGHT, bg="white")
canvas.pack()
# 绘制爱心
t = 0
while True:
# 获取心的坐标
x, y = heart_function(t)
# 绘制心
canvas.create_oval(x, y, x + 10, y + 10, fill=HEART_COLOR, outline=HEART_COLOR)
# 更新参数
t += 0.01
# 更新画布
canvas.update()
# 随机等待一段时间
time.sleep(random.uniform(0.01, 0.1))
# 进入消息循环
root.mainloop()
```
阅读全文
相关推荐
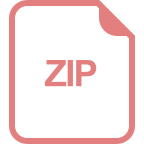
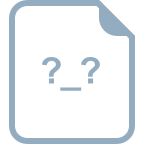
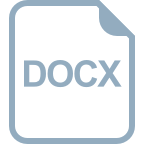
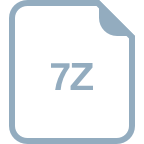
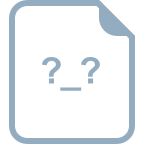
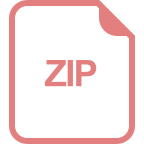
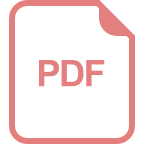
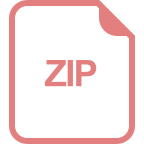
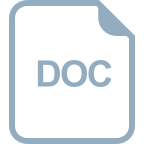
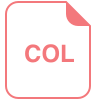






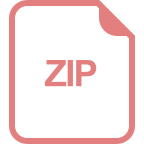