java Overloading
时间: 2023-09-21 12:07:55 浏览: 201
Java Overloading是指在同一个类中,可以定义多个同名的方法,但是这些方法的参数类型或者参数个数不同,从而实现不同的功能。
Java编译器根据调用时传递的参数类型和个数,来决定具体调用哪一个同名方法。这样可以使代码更加简洁,增加代码的可读性和可重用性。
例如,下面的代码演示了两个同名方法add,但是参数列表不同:
```
public class Calculation {
public int add(int x, int y) {
return x + y;
}
public double add(double x, double y) {
return x + y;
}
}
```
在调用add方法时,传递不同类型的参数,Java编译器会自动匹配对应的方法,从而实现不同的功能。
相关问题
java overloading
Java中的overloading是指在同一个类中定义多个同名的方法,但这些方法的参数列表不同。通过使用不同的参数列表来区分方法,使得程序可以根据传入的参数类型或者参数个数选择合适的方法进行调用。
在编写代码时,如果需要执行相似但略有不同的操作,可以使用方法的overloading来简化代码。通过方法的重载,我们可以使用相同的方法名来表示这些操作,提高代码的可读性和可维护性。
overloading in java
In Java, method overloading refers to the ability to define two or more methods with the same name in a class. The methods must have different parameter lists (i.e., different types or different numbers of parameters). This allows us to use the same method name for different behaviors, depending on the type or number of parameters passed to it.
For example, we can define a method called "sum" that takes two integer parameters and returns their sum. We can also define another method called "sum" that takes three integer parameters and returns their sum. When we call the "sum" method, Java will automatically choose the appropriate method based on the number and types of parameters passed to it.
Here's an example of method overloading in Java:
```
public class OverloadingExample {
public static void main(String[] args) {
int a = 5, b = 10, c = 15;
double x = 2.5, y = 3.5;
System.out.println(sum(a, b)); // calls sum(int, int)
System.out.println(sum(a, b, c)); // calls sum(int, int, int)
System.out.println(sum(x, y)); // calls sum(double, double)
}
public static int sum(int a, int b) {
return a + b;
}
public static int sum(int a, int b, int c) {
return a + b + c;
}
public static double sum(double a, double b) {
return a + b;
}
}
```
In this example, we have three "sum" methods with different parameter lists. When we call the "sum" method with two integers, Java will call the first method that takes two integers. When we call the "sum" method with three integers, Java will call the second method that takes three integers. When we call the "sum" method with two doubles, Java will call the third method that takes two doubles.
阅读全文
相关推荐
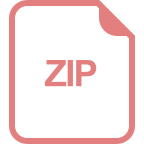
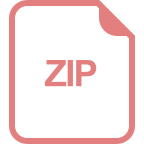
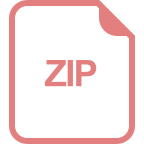
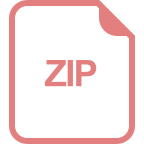
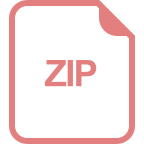

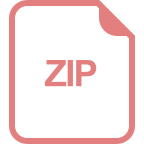
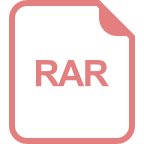
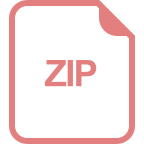
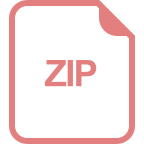
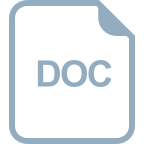
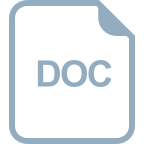


