python图多坐标轴设置坐标轴上的数字的字号twin
时间: 2024-05-12 12:20:35 浏览: 142
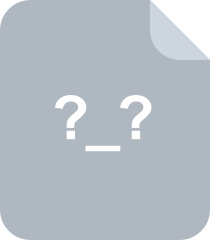
基于python实现设置坐标轴注解
在 Python 中,可以使用 matplotlib 库来设置图多坐标轴,并设置坐标轴上的数字的字号。具体实现方法如下:
1. 导入 matplotlib 库:
```python
import matplotlib.pyplot as plt
```
2. 创建一个图像对象和两个子图:
```python
fig, ax1 = plt.subplots()
ax2 = ax1.twinx()
```
3. 在 ax1 中绘制需要显示在第一个坐标轴上的数据:
```python
ax1.plot(x1, y1, 'r-', label='data1')
```
4. 在 ax2 中绘制需要显示在第二个坐标轴上的数据:
```python
ax2.plot(x2, y2, 'b-', label='data2')
```
5. 设置第一个坐标轴的标签和字号:
```python
ax1.set_xlabel('X Label', fontsize=12)
ax1.set_ylabel('Y1 Label', fontsize=12)
```
6. 设置第二个坐标轴的标签和字号:
```python
ax2.set_ylabel('Y2 Label', fontsize=12)
```
完整示例代码:
```python
import matplotlib.pyplot as plt
import numpy as np
# 创建数据
x1 = np.linspace(0, 10, 100)
y1 = np.sin(x1)
x2 = np.linspace(0, 10, 100)
y2 = np.exp(x2)
# 创建图像对象和两个子图
fig, ax1 = plt.subplots()
ax2 = ax1.twinx()
# 在 ax1 中绘制需要显示在第一个坐标轴上的数据
ax1.plot(x1, y1, 'r-', label='data1')
# 在 ax2 中绘制需要显示在第二个坐标轴上的数据
ax2.plot(x2, y2, 'b-', label='data2')
# 设置第一个坐标轴的标签和字号
ax1.set_xlabel('X Label', fontsize=12)
ax1.set_ylabel('Y1 Label', fontsize=12)
# 设置第二个坐标轴的标签和字号
ax2.set_ylabel('Y2 Label', fontsize=12)
# 显示图例
ax1.legend(loc='upper left')
ax2.legend(loc='upper right')
# 显示图像
plt.show()
```
阅读全文
相关推荐
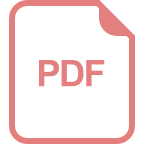
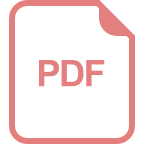















