C语言多文件编写实现单链表的运算
时间: 2024-09-15 20:14:00 浏览: 45
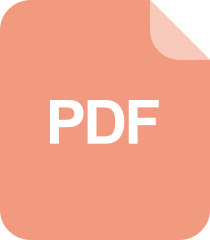
链表的C语言实现之单链表的查找运算
在C语言中,使用多文件编写来实现单链表的运算通常涉及以下几个步骤:
1. 首先,在`list.h`头文件中声明链表结构和基本操作函数的原型,例如节点定义、链表创建、插入节点等:
```c
// list.h
#ifndef LIST_H
#define LIST_H
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* create_list();
void insert_node(Node** head, int value);
void print_list(Node* head);
#endif // LIST_H
```
2. 然后,在`list.c`文件中提供函数的具体实现:
```c
// list.c
#include "list.h"
Node* create_list() {
Node* head = NULL;
return head;
}
void insert_node(Node** head, int value) {
if (*head == NULL) {
*head = (Node*)malloc(sizeof(Node));
(*head)->data = value;
(*head)->next = NULL;
} else {
Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = (Node*)malloc(sizeof(Node));
temp->next->data = value;
temp->next->next = NULL;
}
}
void print_list(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
```
3. 当你在主程序中需要使用链表时,只需包含`list.h`并链接`list.c`文件:
```c
#include <stdio.h>
#include "list.h"
int main() {
Node* head = create_list();
insert_node(&head, 1);
insert_node(&head, 2);
insert_node(&head, 3);
print_list(head);
return 0;
}
```
阅读全文
相关推荐
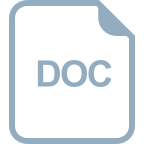
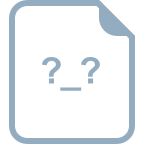
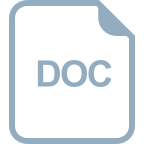




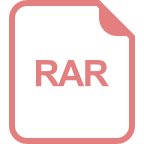
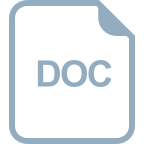
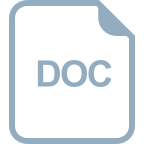







