请用pytorch写出SparseAttention的代码
时间: 2023-08-31 08:20:15 浏览: 55
### 回答1:
好的,以下是使用PyTorch实现SparseAttention的代码:
```
import torch
import torch.nn.functional as F
class SparseAttention(torch.nn.Module):
def __init__(self, num_heads, d_model, dropout=0.1):
super().__init__()
self.num_heads = num_heads
self.d_model = d_model
self.dropout = torch.nn.Dropout(dropout)
self.q_linear = torch.nn.Linear(d_model, d_model, bias=False)
self.k_linear = torch.nn.Linear(d_model, d_model, bias=False)
self.v_linear = torch.nn.Linear(d_model, d_model, bias=False)
self.out_linear = torch.nn.Linear(d_model, d_model, bias=False)
def forward(self, q, k, v, mask=None):
# q, k, and v are of shape (batch_size, seq_len, d_model)
# mask is of shape (batch_size, seq_len)
batch_size = q.size(0)
seq_len = q.size(1)
# Linear projection for the queries, keys, and values
q_proj = self.q_linear(q).view(batch_size, seq_len, self.num_heads, self.d_model // self.num_heads).transpose(1, 2)
k_proj = self.k_linear(k).view(batch_size, seq_len, self.num_heads, self.d_model // self.num_heads).transpose(1, 2)
v_proj = self.v_linear(v).view(batch_size, seq_len, self.num_heads, self.d_model // self.num_heads).transpose(1, 2)
# Compute the attention scores
attn_scores = torch.matmul(q_proj, k_proj.transpose(-2, -1)) / (self.d_model // self.num_heads)**0.5
# Apply the mask
if mask is not None:
attn_scores = attn_scores.masked_fill(mask.unsqueeze(1).unsqueeze(2) == 0, float('-inf'))
# Apply the softmax function
attn_probs = F.softmax(attn_scores, dim=-1)
# Apply the dropout
attn_probs = self.dropout(attn_probs)
# Compute the weighted sum of the values
attn_output = torch.matmul(attn_probs, v_proj)
# Concatenate the heads and apply the output projection
attn_output = attn_output.transpose(1, 2).contiguous().view(batch_size, seq_len, self.num_heads * (self.d_model // self.num_heads))
attn_output = self.out_linear(attn_output)
return attn_output
```
在这个实现中,我们先使用线性投影将输入的queries、keys和values的维度变为d_model,然后将它们分别拆分成num_heads个头,每个头的维度为d_model/num_heads。接着,我们计算每个头的attention得分,然后将它们拼接在一起,再通过一个输出投影层获得最终的attention输出。如果有mask,则在计算attention得分时将mask的位置设置为负无穷,以忽略这些位置的信息。最后,在softmax和输出投影层之前应用dropout以防止过拟合。
### 回答2:
SparseAttention是一种基于稀疏注意力机制的模型,它的PyTorch代码如下所示:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class SparseAttention(nn.Module):
def __init__(self, input_dim, output_dim, sparsity):
super(SparseAttention, self).__init__()
self.input_dim = input_dim
self.output_dim = output_dim
self.sparsity = sparsity
# 初始化参数
self.weights = nn.Parameter(torch.Tensor(input_dim, output_dim))
self.bias = nn.Parameter(torch.Tensor(output_dim))
self.reset_parameters()
def reset_parameters(self):
nn.init.xavier_uniform_(self.weights)
nn.init.zeros_(self.bias)
def forward(self, x):
# 特征投影
projected = torch.matmul(x, self.weights)
# 计算注意力分数
attention_scores = F.softmax(projected, dim=-1)
# 获取稀疏的注意力分数
num_sparse = int(self.sparsity * self.output_dim)
_, top_indices = torch.topk(attention_scores, num_sparse, dim=-1)
sparse_attention_scores = torch.zeros_like(attention_scores)
sparse_attention_scores.scatter_(-1, top_indices, attention_scores.gather(-1, top_indices))
# 加权求和
weighted = torch.matmul(sparse_attention_scores, projected.transpose(-1, -2))
# 添加偏置
output = weighted + self.bias
return output
```
以上的代码实现了SparseAttention模型,其中`input_dim`表示输入的特征维度,`output_dim`表示输出的特征维度,`sparsity`表示稀疏比例。在前向传播过程中,首先对输入特征进行线性投影,然后计算所有注意力分数,并根据稀疏比例选择出topk的注意力分数。接着,将稀疏的注意力分数与投影特征进行加权求和,并添加偏置。最终得到输出的特征。注意,上述实现仅供参考,具体使用时需要根据实际情况进行调整。
### 回答3:
SparseAttention是一种特殊类型的注意力机制,用于处理稀疏输入数据。在PyTorch中,我们可以使用以下代码实现SparseAttention。
首先,我们需要导入PyTorch库和其他相关库:
```python
import torch
import torch.nn as nn
```
然后,我们可以定义SparseAttention类,并继承PyTorch的nn.Module类:
```python
class SparseAttention(nn.Module):
def __init__(self, input_dim, hidden_dim):
super(SparseAttention, self).__init__()
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.fc = nn.Linear(input_dim, hidden_dim)
def forward(self, input):
# 线性变换
hidden = self.fc(input)
# 计算注意力权重
attn_weights = torch.softmax(hidden, dim=-1)
# 计算加权平均向量
weighted_input = torch.sum(input * attn_weights.unsqueeze(-1), dim=-2)
return weighted_input
```
我们在SparseAttention类的构造函数中定义了输入维度(input_dim)和隐藏维度(hidden_dim)。在forward方法中,我们首先对输入数据进行线性变换,然后使用softmax函数计算注意力权重,并将输入与注意力权重相乘。最后,我们通过对注意力加权输入进行求和操作,得到加权平均向量。
接下来,我们可以创建SparseAttention的实例,并将输入数据传递给它:
```python
input_dim = 10
hidden_dim = 5
input = torch.randn(3, 5, input_dim) # 生成3个输入数据,每个数据包含5个特征
sparse_attention = SparseAttention(input_dim, hidden_dim)
output = sparse_attention(input)
print(output)
```
在这个例子中,我们创建了一个大小为3x5xinput_dim的输入数据。然后,我们创建了一个SparseAttention实例并将输入数据传递给它。最后,我们打印输出结果output。
这就是用PyTorch实现SparseAttention的代码。希望对你有所帮助!
相关推荐
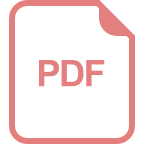





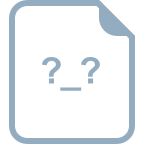
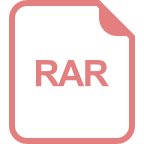
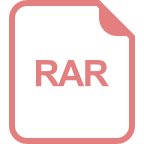
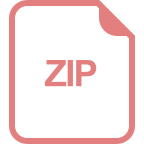
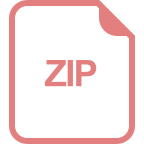
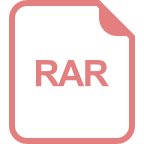
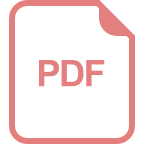
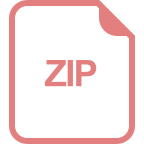
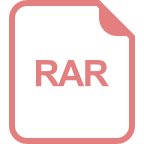
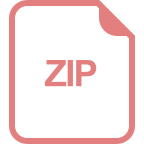