请帮我用pytorch写出SparseAttention的代码
时间: 2024-02-11 13:09:38 浏览: 86
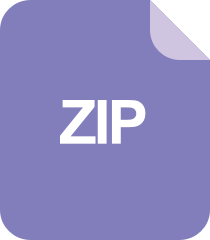
pytorch手写数字识别代码.zip
好的,以下是一个简单的SparseAttention的代码实现示例:
```python
import torch
import torch.nn as nn
class SparseAttention(nn.Module):
def __init__(self, input_size, output_size, num_heads, dropout=0.1):
super(SparseAttention, self).__init__()
self.num_heads = num_heads
self.head_size = output_size // num_heads
self.query = nn.Linear(input_size, output_size)
self.key = nn.Linear(input_size, output_size)
self.value = nn.Linear(input_size, output_size)
self.dropout = nn.Dropout(dropout)
self.output = nn.Linear(output_size, output_size)
def forward(self, inputs):
batch_size, seq_len, input_size = inputs.size()
# Compute query, key, and value
q = self.query(inputs)
k = self.key(inputs)
v = self.value(inputs)
# Reshape query, key, and value for multi-head attention
q = q.view(batch_size, seq_len, self.num_heads, self.head_size)
k = k.view(batch_size, seq_len, self.num_heads, self.head_size)
v = v.view(batch_size, seq_len, self.num_heads, self.head_size)
# Compute attention scores
scores = torch.matmul(q, k.transpose(-2, -1)) / self.head_size**0.5
# Compute sparse attention scores
mask = torch.ones(batch_size, seq_len, seq_len)
for i in range(seq_len):
start = max(0, i - 2)
end = min(seq_len, i + 3)
mask[:, i, start:end] = 0
scores.masked_fill_(mask == 1, float('-inf'))
# Apply softmax to get attention probabilities
attn_probs = nn.functional.softmax(scores, dim=-1)
attn_probs = self.dropout(attn_probs)
# Apply attention to value
attn_output = torch.matmul(attn_probs, v)
attn_output = attn_output.view(batch_size, seq_len, self.num_heads * self.head_size)
# Apply output layer
output = self.output(attn_output)
output = self.dropout(output)
return output
```
其中,`SparseAttention`类包含了一个稀疏注意力机制,输入为一个三维张量`(batch_size, seq_len, input_size)`,输出为一个三维张量`(batch_size, seq_len, output_size)`。具体实现过程如下:
1. 首先,使用三个线性层(`query`、`key`和`value`)将输入转换为查询、键和值。
2. 将查询、键和值分别重塑为`(batch_size, seq_len, num_heads, head_size)`的形状,以进行多头注意力计算。
3. 计算注意力得分,并使用一个掩码矩阵来实现稀疏注意力机制。该掩码矩阵是一个形状为`(batch_size, seq_len, seq_len)`的张量,其中每个元素为0或1,表示是否需要忽略对应位置的元素。在本例中,掩码矩阵的每一行只允许与当前位置距离不超过2的元素互动,从而实现了稀疏注意力。
4. 将注意力得分应用softmax函数,得到注意力概率,并使用dropout进行正则化。
5. 使用注意力概率和值计算注意力输出,并将其重塑为`(batch_size, seq_len, output_size)`的形状。
6. 最后,使用一个线性层和dropout进行最终输出。
阅读全文
相关推荐





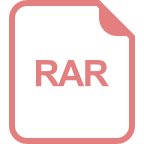
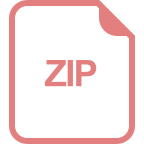
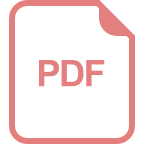
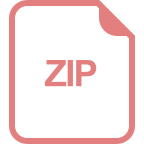
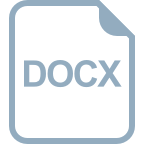
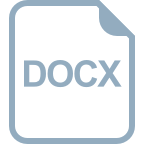
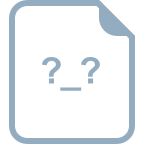
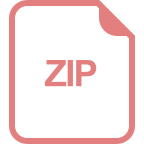
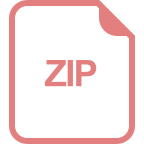
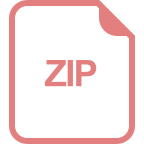