<?php include "function.php"; $process = shell_exec("ps aux | grep 'ntpd' | grep -v 'grep'"); if($process){ echo 'NTP服务正在运行'; }else{ echo 'NTP服务没有运行'; } //指定文件路径和文件名 $file_path = "/etc/ntp.conf"; //判断是否收到了保存文件的请求 if(isset($_POST['content'])){ //获取客户端传来的文件内容 $content = $_POST['content']; //打开文件并进行写入操作 $handle = fopen($file_path,"w"); fwrite($handle,$content); fclose($handle); //返回成功的信息给客户端 echo json_encode(array("status" => "success")); exit; } //打开文件并读取文件内容 $handle = fopen($file_path,"r"); $content = fread($handle,filesize($file_path)); fclose($handle); ?> html用ajax调用该代码 实现两个按钮 一个可以打开和编辑文件 一个可以保存和传回到被文件所在的路径的完整代码
时间: 2023-08-13 12:03:50 浏览: 52
以下是可以实现你所需功能的完整代码:
index.html文件
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>编辑NTP配置文件</title>
<script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<h1>编辑NTP配置文件</h1>
<hr>
<button onclick="editFile()">编辑文件</button>
<button onclick="saveFile()">保存文件</button>
<br><br>
<textarea id="fileContent" cols="80" rows="30"></textarea>
<script>
function editFile() {
$.ajax({
url: "edit.php",
type: "GET",
success: function (response) {
$("#fileContent").val(response);
}
});
}
function saveFile() {
var fileContent = $("#fileContent").val();
$.ajax({
url: "edit.php",
type: "POST",
data: {content: fileContent},
dataType: "json",
success: function (response) {
if (response.status == "success") {
alert("文件保存成功!");
}
}
});
}
</script>
</body>
</html>
```
edit.php文件
```
<?php
include "function.php";
$process = shell_exec("ps aux | grep 'ntpd' | grep -v 'grep'");
if ($process) {
echo 'NTP服务正在运行<br>';
} else {
echo 'NTP服务没有运行<br>';
}
//指定文件路径和文件名
$file_path = "/etc/ntp.conf";
//判断是否收到了保存文件的请求
if (isset($_POST['content'])) {
//获取客户端传来的文件内容
$content = $_POST['content'];
//打开文件并进行写入操作
$handle = fopen($file_path, "w");
fwrite($handle, $content);
fclose($handle);
//返回成功的信息给客户端
echo json_encode(array("status" => "success"));
exit;
}
//打开文件并读取文件内容
$handle = fopen($file_path, "r");
$content = fread($handle, filesize($file_path));
fclose($handle);
echo $content;
?>
```
function.php文件
```
<?php
//此文件中可以放置一些公共的函数和常量定义
?>
```
需要注意的是,这段代码需要运行在具备访问/etc/ntp.conf文件和执行shell命令的权限的服务器上。
相关推荐
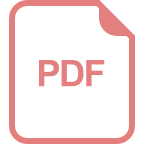
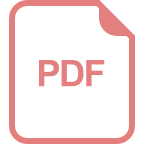
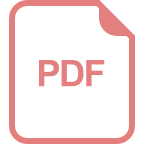











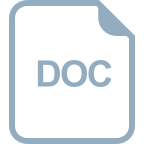
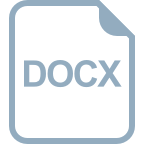
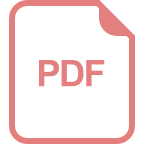
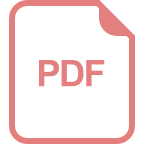