ga-lstm代码
时间: 2023-07-02 08:08:47 浏览: 138
以下是一个简单的 GA-LSTM 模型的 Python 代码示例:
```python
import numpy as np
import random
class GALSTM():
def __init__(self, input_size, output_size, hidden_size, population_size, mutation_rate):
self.input_size = input_size
self.output_size = output_size
self.hidden_size = hidden_size
self.population_size = population_size
self.mutation_rate = mutation_rate
self.population = []
self.fitness_scores = []
# Initialize population
for i in range(population_size):
self.population.append(self.generate_individual())
def generate_individual(self):
# Generate weights for the GA-LSTM individual
# Each individual is a numpy array with shape (input_size + hidden_size + 1, 4 * hidden_size + output_size)
individual = np.zeros((self.input_size + self.hidden_size + 1, 4 * self.hidden_size + self.output_size))
for i in range(self.input_size + self.hidden_size + 1):
for j in range(4 * self.hidden_size + self.output_size):
individual[i][j] = random.uniform(-1, 1)
return individual
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def forward(self, x, h, c, individual):
# Perform forward pass through the GA-LSTM individual
# x is the input vector, h is the previous hidden state, c is the previous cell state
# individual is the numpy array representing the weights of the individual
input_concat = np.concatenate((x, h, np.array([1])))
gates = np.dot(input_concat, individual)
input_gate = self.sigmoid(gates[:self.hidden_size])
forget_gate = self.sigmoid(gates[self.hidden_size:2*self.hidden_size])
output_gate = self.sigmoid(gates[2*self.hidden_size:3*self.hidden_size])
cell_update = np.tanh(gates[3*self.hidden_size:4*self.hidden_size])
c_new = c * forget_gate + input_gate * cell_update
h_new = output_gate * np.tanh(c_new)
return h_new, c_new
def predict(self, x, individual):
# Use the GA-LSTM individual to make a prediction for the input x
h = np.zeros(self.hidden_size)
c = np.zeros(self.hidden_size)
for i in range(len(x)):
h, c = self.forward(x[i], h, c, individual)
output = np.dot(np.concatenate((h, np.array([1]))), individual)[-self.output_size:]
return output
def evaluate_fitness(self, x_train, y_train, individual):
# Evaluate the fitness of the GA-LSTM individual using mean squared error
mse = 0
for i in range(len(x_train)):
y_pred = self.predict(x_train[i], individual)
mse += np.mean((y_pred - y_train[i])**2)
fitness = 1 / (mse + 1e-6)
return fitness
def select_parents(self):
# Select two parents from the population using tournament selection
parent1, parent2 = None, None
for i in range(2):
tournament_indices = np.random.choice(self.population_size, size=5, replace=False)
tournament_individuals = [self.population[i] for i in tournament_indices]
tournament_fitness_scores = [self.fitness_scores[i] for i in tournament_indices]
winner = tournament_individuals[np.argmax(tournament_fitness_scores)]
if i == 0:
parent1 = winner
else:
parent2 = winner
return parent1, parent2
def crossover(self, parent1, parent2):
# Perform crossover between two parents to create a new child
child = np.zeros(parent1.shape)
for i in range(parent1.shape[0]):
for j in range(parent1.shape[1]):
if random.random() < 0.5:
child[i][j] = parent1[i][j]
else:
child[i][j] = parent2[i][j]
return child
def mutate(self, child):
# Perform mutation on a child
for i in range(child.shape[0]):
for j in range(child.shape[1]):
if random.random() < self.mutation_rate:
child[i][j] += random.uniform(-1, 1)
return child
def evolve(self, x_train, y_train):
# Evolve the population using genetic algorithm
self.fitness_scores = [self.evaluate_fitness(x_train, y_train, individual) for individual in self.population]
sorted_indices = np.argsort(self.fitness_scores)[::-1]
self.population = [self.population[i] for i in sorted_indices]
elite_individual = self.population[0]
new_population = [elite_individual]
for i in range(1, self.population_size):
parent1, parent2 = self.select_parents()
child = self.crossover(parent1, parent2)
child = self.mutate(child)
new_population.append(child)
self.population = new_population
def fit(self, x_train, y_train, num_generations):
# Train the GA-LSTM model on the training data for a given number of generations
for i in range(num_generations):
self.evolve(x_train, y_train)
fitness_scores = [self.evaluate_fitness(x_train, y_train, individual) for individual in self.population]
print("Generation", i+1, "- Best Fitness:", max(fitness_scores))
return self.population[0]
```
这个 GA-LSTM 类包括以下方法:
- `__init__(self, input_size, output_size, hidden_size, population_size, mutation_rate)`:初始化 GA-LSTM 模型,其中`input_size`是输入向量的维数,`output_size`是输出向量的维数,`hidden_size`是隐藏层向量的维数,`population_size`是种群大小,`mutation_rate`是突变率。
- `generate_individual(self)`:生成 GA-LSTM 个体的权重矩阵。
- `sigmoid(self, x)`:计算 sigmoid 函数的值。
- `forward(self, x, h, c, individual)`:执行 GA-LSTM 个体的前向传播。
- `predict(self, x, individual)`:使用 GA-LSTM 个体对输入 x 进行预测。
- `evaluate_fitness(self, x_train, y_train, individual)`:计算 GA-LSTM 个体的适应度分数。
- `select_parents(self)`:使用锦标赛选择法从种群中选择两个父代。
- `crossover(self, parent1, parent2)`:在两个父代之间执行交叉操作以创建一个新的孩子。
- `mutate(self, child)`:对一个孩子执行突变操作。
- `evolve(self, x_train, y_train)`:使用遗传算法进化种群。
- `fit(self, x_train, y_train, num_generations)`:在训练数据上训练 GA-LSTM 模型。
阅读全文
相关推荐
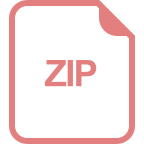
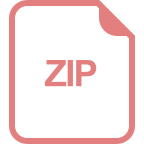
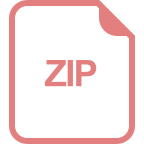
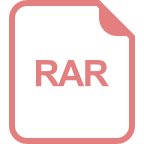
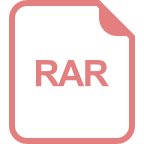
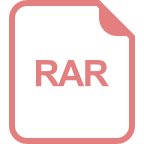
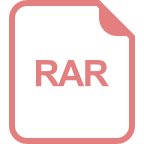
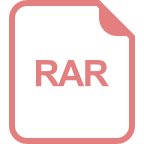
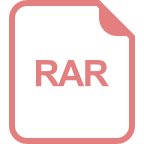
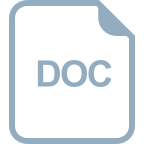
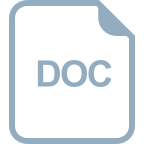
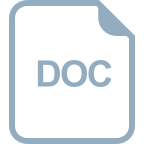
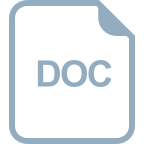



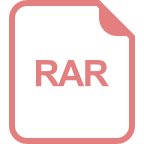
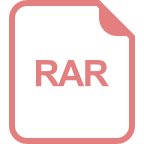