java中redis set 的value是一个字符串,怎么序列化成一个对象然后进行set
时间: 2024-03-01 16:55:57 浏览: 20
在 Java 中,如果要将一个对象序列化为字符串后再存储到 Redis 中,可以使用一些常见的序列化工具,比如 JSON、Jackson、Protobuf 等,将对象转换为字符串后再进行存储。
以下是将一个 Java 对象使用 Jackson 序列化为 JSON 字符串,并使用 Jedis 客户端进行存储的示例:
```java
import com.fasterxml.jackson.databind.ObjectMapper;
import redis.clients.jedis.Jedis;
public class RedisExample {
public static void main(String[] args) throws Exception {
// 创建 Redis 客户端连接
Jedis jedis = new Jedis("localhost");
// 定义一个 Java 对象
User user = new User("Alice", 25, "alice@example.com");
// 使用 Jackson 序列化为 JSON 字符串
ObjectMapper mapper = new ObjectMapper();
String userJson = mapper.writeValueAsString(user);
// 将序列化后的字符串保存到 Redis 中
jedis.set("user:1", userJson);
// 关闭 Redis 客户端连接
jedis.close();
}
}
class User {
private String name;
private int age;
private String email;
public User(String name, int age, String email) {
this.name = name;
this.age = age;
this.email = email;
}
// getter 和 setter 方法省略
}
```
在这个示例中,我们使用 Jackson 序列化工具将一个 Java 对象序列化为 JSON 字符串,并使用 Jedis 客户端的 `set` 方法将其保存到 Redis 中。在读取数据时,需要将序列化后的字符串反序列化为 Java 对象。以下是从 Redis 中读取并反序列化为 Java 对象的示例:
```java
import com.fasterxml.jackson.databind.ObjectMapper;
import redis.clients.jedis.Jedis;
public class RedisExample {
public static void main(String[] args) throws Exception {
// 创建 Redis 客户端连接
Jedis jedis = new Jedis("localhost");
// 从 Redis 中读取序列化后的字符串
String userJson = jedis.get("user:1");
// 使用 Jackson 反序列化为 Java 对象
ObjectMapper mapper = new ObjectMapper();
User user = mapper.readValue(userJson, User.class);
// 输出用户信息
System.out.println(user.getName()); // Alice
System.out.println(user.getAge()); // 25
System.out.println(user.getEmail()); // alice@example.com
// 关闭 Redis 客户端连接
jedis.close();
}
}
class User {
private String name;
private int age;
private String email;
public User() {
}
public User(String name, int age, String email) {
this.name = name;
this.age = age;
this.email = email;
}
// getter 和 setter 方法省略
}
```
在这个示例中,我们使用 Jedis 客户端的 `get` 方法从 Redis 中读取序列化后的字符串,然后使用 Jackson 反序列化工具将其反序列化为 Java 对象,并输出用户信息。
相关推荐
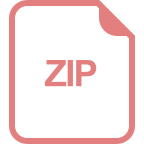
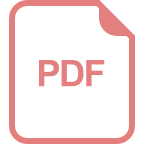
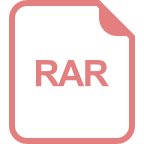














