使用socket封装一个java操作redis的工具类
时间: 2023-11-27 21:50:38 浏览: 33
好的,以下是一个简单的使用Socket封装的Java操作Redis的工具类:
```java
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.Socket;
import java.util.ArrayList;
import java.util.List;
public class RedisUtils {
private static final String HOST = "127.0.0.1";
private static final int PORT = 6379;
private Socket socket;
private InputStream input;
private OutputStream output;
public RedisUtils() {
try {
this.socket = new Socket(HOST, PORT);
this.input = socket.getInputStream();
this.output = socket.getOutputStream();
} catch (IOException e) {
e.printStackTrace();
}
}
public String set(String key, String value) {
String result = null;
try {
output.write(("SET " + key + " " + value + "\r\n").getBytes());
byte[] bytes = new byte[1024];
int length = input.read(bytes);
result = new String(bytes, 0, length);
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
public String get(String key) {
String result = null;
try {
output.write(("GET " + key + "\r\n").getBytes());
byte[] bytes = new byte[1024];
int length = input.read(bytes);
result = new String(bytes, 0, length);
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
public List<String> lrange(String key, int start, int end) {
List<String> result = new ArrayList<>();
try {
output.write(("LRANGE " + key + " " + start + " " + end + "\r\n").getBytes());
byte[] bytes = new byte[1024];
int length = input.read(bytes);
String str = new String(bytes, 0, length);
if (str.startsWith("*")) {
int count = Integer.parseInt(str.substring(1, str.indexOf("\r\n")));
for (int i = 0; i < count; i++) {
length = input.read(bytes);
result.add(new String(bytes, 0, length).trim());
}
}
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
public void close() {
try {
input.close();
output.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这个工具类实现了Redis的三个基本操作:SET、GET和LRANGE。其中,SET和GET操作都是将字符串拼接成Redis协议格式,然后通过Socket发送给Redis服务端,最后接收Redis服务端返回的数据,转换成Java字符串格式并返回。LRANGE操作稍微复杂一些,因为需要接收Redis服务端返回的多个字符串,所以需要对返回的数据进行解析。最后,还提供了一个close方法,用于关闭Socket连接。
相关推荐
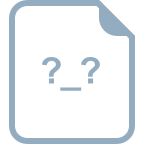














