使用socket封装一个java可执行redis命令的工具类
时间: 2023-11-27 16:50:42 浏览: 65
以下是一个简单的Java工具类,用于连接到Redis服务器并执行命令:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.Socket;
public class RedisClient {
private String host;
private int port;
private Socket socket;
private BufferedReader input;
private PrintWriter output;
public RedisClient(String host, int port) {
this.host = host;
this.port = port;
}
public void connect() throws Exception {
socket = new Socket(host, port);
input = new BufferedReader(new InputStreamReader(socket.getInputStream()));
output = new PrintWriter(socket.getOutputStream(), true);
}
public void sendCommand(String command) throws Exception {
output.println(command);
String response = input.readLine();
System.out.println(response);
}
public void disconnect() throws Exception {
input.close();
output.close();
socket.close();
}
}
```
使用该工具类可以执行Redis命令,例如:
```java
public static void main(String[] args) {
try {
RedisClient client = new RedisClient("localhost", 6379);
client.connect();
client.sendCommand("SET mykey somevalue");
client.sendCommand("GET mykey");
client.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
```
这个例子展示了如何连接到本地Redis服务器,设置一个键值对,并获取该键的值。你可以根据需要修改或扩展该工具类来执行其他Redis命令。
阅读全文
相关推荐
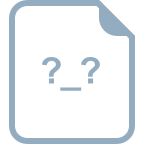




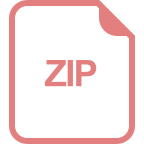
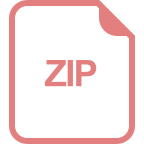
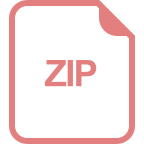
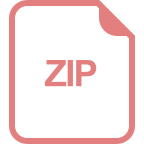
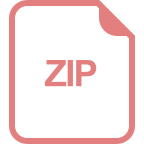
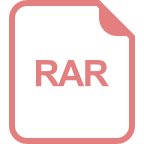
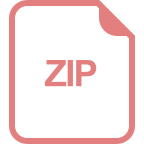
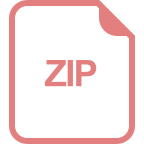
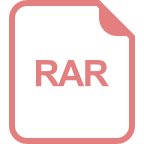
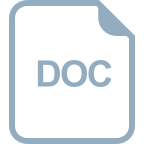
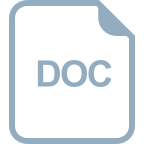
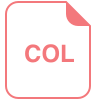