用python编写银行取款代码
时间: 2023-07-23 22:50:13 浏览: 129
以下是一个简单的Python代码示例,用于模拟银行取款操作:
```python
# 定义初始余额
balance = 10000
# 定义取款函数
def withdraw(amount):
# 声明全局变量
global balance
# 判断余额是否充足
if balance >= amount:
balance -= amount
print("取款成功!当前余额为:", balance)
else:
print("余额不足,取款失败!当前余额为:", balance)
# 测试取款功能
withdraw(2000) # 取出2000元
withdraw(8000) # 取出8000元
withdraw(5000) # 取出5000元
```
在上面的代码中,我们定义了一个初始余额为10000元的变量`balance`,并定义了一个取款函数`withdraw`。该函数接受一个参数`amount`表示取款金额,如果余额充足,则从余额中扣除对应的金额,并输出取款成功的信息和当前余额;否则输出余额不足的信息和当前余额。最后我们测试了三次取款操作,分别取出2000元、8000元和5000元。你可以根据需要进行修改和完善。
相关问题
用Python编写银行系统
以下是一个简单的Python银行系统的示例代码,包括开户、查询、存款、取款、转账、改密、冻结、解冻、补卡和销户等功能:
```python
class Bank:
def __init__(self, name, balance=0):
self.name = name
self.balance = balance
self.status = 'active'
def deposit(self, amount):
if self.status == 'active':
self.balance += amount
print(f'Deposit successful. Current balance: {self.balance}')
else:
print('Your account is frozen. Please contact customer service.')
def withdraw(self, amount):
if self.status == 'active':
if amount > self.balance:
print('Insufficient balance.')
else:
self.balance -= amount
print(f'Withdrawal successful. Current balance: {self.balance}')
else:
print('Your account is frozen. Please contact customer service.')
def transfer(self, amount, recipient):
if self.status == 'active':
if amount > self.balance:
print('Insufficient balance.')
else:
self.balance -= amount
recipient.balance += amount
print(f'Transfer successful. Current balance: {self.balance}')
else:
print('Your account is frozen. Please contact customer service.')
def freeze(self):
self.status = 'frozen'
print('Your account has been frozen.')
def unfreeze(self):
self.status = 'active'
print('Your account has been unfrozen.')
def change_password(self, new_password):
self.password = new_password
print('Password changed successfully.')
def close_account(self):
self.status = 'closed'
print('Your account has been closed.')
def __str__(self):
return f'Account name: {self.name}\nBalance: {self.balance}\nStatus: {self.status}'
# 示例代码
account1 = Bank('Alice', 1000)
account2 = Bank('Bob', 500)
account1.deposit(500)
account1.withdraw(200)
account1.transfer(300, account2)
print(account1)
print(account2)
```
使用python编写一个简单的银行代码
好的,我们可以创建一个简单的Python银行账户类(BankAccount),它包含基本的存款(deposit)、取款(withdraw)和余额查询(balance_check)功能。这个例子不会涉及到复杂的安全机制,仅用于展示基本的面向对象编程概念。
```python
class BankAccount:
def __init__(self, account_number, initial_balance=0):
self.account_number = account_number
self.balance = initial_balance
# 存款方法
def deposit(self, amount):
if amount > 0:
self.balance += amount
print(f"已存入 {amount} 元,当前余额为 {self.balance} 元")
else:
print("请输入正数作为存款金额。")
# 取款方法
def withdraw(self, amount):
if amount <= self.balance:
self.balance -= amount
print(f"已提取 {amount} 元,当前余额为 {self.balance} 元")
elif amount > self.balance:
print("余额不足,无法完成取款操作。")
else:
print("请输入有效的取款金额。")
# 查询余额
def balance_check(self):
return f"您的当前余额为 {self.balance} 元"
# 示例使用
account = BankAccount(123456789)
account.deposit(5000)
account.withdraw(2000)
print(account.balance_check())
```
阅读全文
相关推荐
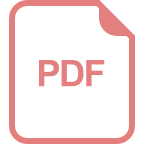
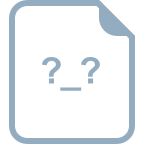
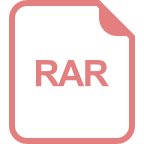
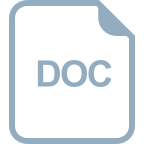




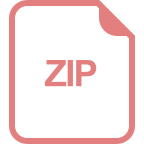
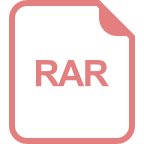
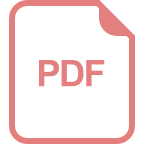
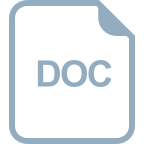
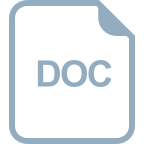
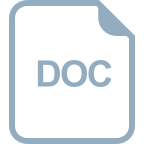

