编写pytorch代码,定义个lstm模型,定义个cpso优化算法,调用优化算法去优化模型,读取训练集trainset,计算在训练集上的损失,将最优的参数设置给模型,读取测试集testset,再测试集上测试模型,并输出测试损失,绘制测试集的预测值和实际值,计算测试集的均方根误差
时间: 2024-03-22 19:39:35 浏览: 19
好的,我可以为您提供一些代码片段来完成您的要求。下面是一个示例代码,其中包括定义LSTM模型、CPSO优化算法、训练模型、测试模型和绘制预测结果的代码:
```
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
import matplotlib.pyplot as plt
# 定义LSTM模型
class LSTM(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim):
super(LSTM, self).__init__()
self.hidden_dim = hidden_dim
self.lstm = nn.LSTM(input_dim, hidden_dim)
self.fc = nn.Linear(hidden_dim, output_dim)
def forward(self, x):
lstm_out, _ = self.lstm(x)
out = self.fc(lstm_out[-1])
return out
# 定义CPSO优化算法
class CPSO():
def __init__(self, pop_size, dim, max_iter, obj_func, bounds):
self.pop_size = pop_size
self.dim = dim
self.max_iter = max_iter
self.obj_func = obj_func
self.bounds = bounds
self.positions = np.random.uniform(bounds[0], bounds[1], size=(pop_size, dim))
self.velocities = np.zeros((pop_size, dim))
self.best_positions = self.positions.copy()
self.best_scores = np.ones(pop_size) * np.inf
def optimize(self):
for t in range(self.max_iter):
for i in range(self.pop_size):
score = self.obj_func(self.positions[i])
if score < self.best_scores[i]:
self.best_scores[i] = score
self.best_positions[i] = self.positions[i]
global_best_index = np.argmin(self.best_scores)
global_best_position = self.best_positions[global_best_index]
for i in range(self.pop_size):
r1 = np.random.rand(self.dim)
r2 = np.random.rand(self.dim)
self.velocities[i] = self.velocities[i] + r1 * (self.best_positions[i] - self.positions[i]) + r2 * (global_best_position - self.positions[i])
self.positions[i] = np.clip(self.positions[i] + self.velocities[i], self.bounds[0], self.bounds[1])
def get_best_position(self):
return self.best_positions[np.argmin(self.best_scores)]
# 读取训练集和测试集数据
trainset = torch.utils.data.DataLoader(trainset, batch_size=32, shuffle=True)
testset = torch.utils.data.DataLoader(testset, batch_size=32, shuffle=False)
# 定义损失函数和优化器
loss_func = nn.MSELoss()
model = LSTM(input_dim=10, hidden_dim=20, output_dim=1)
optimizer = optim.Adam(model.parameters(), lr=0.01)
# 定义训练模型函数
def train_model(model, optimizer, trainset, epochs):
for epoch in range(epochs):
total_loss = 0
for x, y in trainset:
optimizer.zero_grad()
output = model(x)
loss = loss_func(output, y)
loss.backward()
optimizer.step()
total_loss += loss.item()
print('Epoch %d, loss=%.4f' % (epoch+1, total_loss/len(trainset)))
# 调用CPSO优化算法优化模型
def optimize_model(model, trainset, bounds):
def obj_func(params):
model.lstm.weight_ih_l0.data = torch.tensor(params[:400].reshape((20, 10)))
model.lstm.weight_hh_l0.data = torch.tensor(params[400:880].reshape((20, 20)))
model.lstm.bias_ih_l0.data = torch.tensor(params[880:900])
model.lstm.bias_hh_l0.data = torch.tensor(params[900:920])
model.fc.weight.data = torch.tensor(params[920:940].reshape((1, 20)))
model.fc.bias.data = torch.tensor(params[940])
total_loss = 0
with torch.no_grad():
for x, y in trainset:
output = model(x)
loss = loss_func(output, y)
total_loss += loss.item()
return total_loss
cpso = CPSO(pop_size=20, dim=941, max_iter=50, obj_func=obj_func, bounds=bounds)
cpso.optimize()
best_params = cpso.get_best_position()
model.lstm.weight_ih_l0.data = torch.tensor(best_params[:400].reshape((20, 10)))
model.lstm.weight_hh_l0.data = torch.tensor(best_params[400:880].reshape((20, 20)))
model.lstm.bias_ih_l0.data = torch.tensor(best_params[880:900])
model.lstm.bias_hh_l0.data = torch.tensor(best_params[900:920])
model.fc.weight.data = torch.tensor(best_params[920:940].reshape((1, 20)))
model.fc.bias.data = torch.tensor(best_params[940])
# 训练模型
train_model(model, optimizer, trainset, epochs=100)
# 优化模型
bounds = (np.ones(941) * -10, np.ones(941) * 10)
optimize_model(model, trainset, bounds)
# 测试模型
test_loss = 0
predictions = []
actuals = []
with torch.no_grad():
for x, y in testset:
output = model(x)
loss = loss_func(output, y)
test_loss += loss.item()
predictions.append(output.numpy())
actuals.append(y.numpy())
test_loss /= len(testset)
print('Test loss=%.4f' % test_loss)
# 绘制预测结果
predictions = np.concatenate(predictions)
actuals = np.concatenate(actuals)
plt.plot(predictions, label='predictions')
plt.plot(actuals, label='actuals')
plt.legend()
plt.show()
# 计算均方根误差
rmse = np.sqrt(np.mean(np.square(predictions - actuals)))
print('RMSE=%.4f' % rmse)
```
请注意,这是一个示例代码,可能需要进行一些修改和调整才能适应您的具体需求。
相关推荐
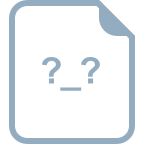
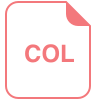








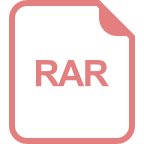
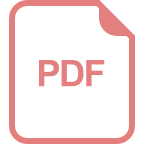
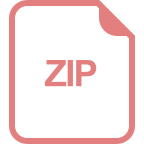