np_utils.to_categorical(y_train)
时间: 2024-01-21 08:19:28 浏览: 26
这是 Keras 中的一个函数,用于将一维的类别标签向量转换成独热编码矩阵。具体来说,假设 y_train 是一个长度为 n 的一维数组,取值范围为 0 到 num_classes - 1,那么 np_utils.to_categorical(y_train) 将返回一个形状为 (n, num_classes) 的二维数组,每一行表示一个样本的独热编码。例如,如果 y_train = [0, 2, 1, 1, 0] 且 num_classes = 3,则转换后的结果为:
```
[[1. 0. 0.]
[0. 0. 1.]
[0. 1. 0.]
[0. 1. 0.]
[1. 0. 0.]]
```
其中每一行表示一个样本的独热编码。
相关问题
y_train = np_utils.to_categorical(y_train, 10) y_test = np_utils.to_categorical(y_test, 10)是什么意思
这是将分类标签转换为one-hot编码的代码,其中:
- `y_train` 和 `y_test` 是样本的分类标签,每个标签是一个整数值,表示该样本属于哪个类别。
- `np_utils.to_categorical` 是 Keras 中的一个实用函数,用于将整数型的类别标签转换为 one-hot 编码形式。
- `10` 是类别总数,即分类问题中的类别数目,这里假设有 10 个类别。
举个例子,如果有一组分类问题的标签如下:
```
[0, 3, 2, 1, 4, 2, 3, 1, 0, 4]
```
那么经过 `np_utils.to_categorical` 转换之后,得到的结果将是一个形状为 `(10, 5)` 的矩阵,其中每一行表示一个标签,每个标签被转换为一个长度为 5 的 one-hot 向量,如下所示:
```
[[1. 0. 0. 0. 0.]
[0. 0. 0. 1. 0.]
[0. 0. 1. 0. 0.]
[0. 1. 0. 0. 0.]
[0. 0. 0. 0. 1.]
[0. 0. 1. 0. 0.]
[0. 0. 0. 1. 0.]
[0. 1. 0. 0. 0.]
[1. 0. 0. 0. 0.]
[0. 0. 0. 0. 1.]]
```
这样做的目的是为了让机器学习模型更好地理解和处理分类问题。
Unused import statement 'from keras.utils.np_utils import to_categorical'
这个错误信息表示你的代码中导入了 `from keras.utils.np_utils import to_categorical` 这个模块,但是并没有在代码中使用到它。这样的导入语句会增加代码的复杂度和运行时间,因此建议将其删除。
如果你确实需要使用 `to_categorical` 这个函数,那么你可以在代码中调用它,例如:
```python
from keras.utils.np_utils import to_categorical
# 将标签转换为 one-hot 编码
y_train = to_categorical(y_train, num_classes=10)
```
这样就不会出现上述的警告信息了。
相关推荐
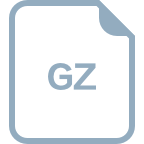
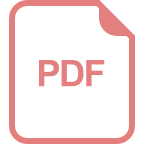
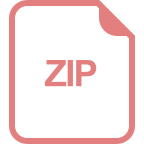












