请用c语言完成任意给定一个有向图,设计一个算法,对它进行拓扑排序。拓扑排序算法思想:a.在有向图中任选一个没有前趋的顶点输出;b.从图中删除该顶点和所有以它为尾的弧;c.重复上述a、b,直到全部顶点都已输出,此时,顶点输出序列即为一个拓朴有序序列;或者直到图中没有无前趋的顶点为止,此情形表明有向图中存在环。
时间: 2024-02-28 17:56:54 浏览: 23
好的,我可以为您提供一个基于邻接表的有向图拓扑排序的C语言实现。首先,我们需要定义一个结构体来表示有向图中的每个节点,如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTEX_NUM 100 // 最大节点数
// 节点结构体
typedef struct node {
int data; // 节点数据
struct node *next; // 指向下一个节点的指针
} Node;
// 邻接表结构体
typedef struct graph {
Node *vertices[MAX_VERTEX_NUM]; // 节点数组
int indegree[MAX_VERTEX_NUM]; // 节点入度数组
int vertex_num; // 节点数目
} Graph;
```
在定义好节点结构体和邻接表结构体后,我们需要实现一个函数来创建有向图,并且初始化每个节点的入度为0,如下所示:
```c
// 创建有向图
Graph* create_graph(int vertex_num) {
Graph *graph = (Graph*)malloc(sizeof(Graph));
graph->vertex_num = vertex_num;
for (int i = 0; i < vertex_num; i++) {
graph->vertices[i] = NULL;
graph->indegree[i] = 0;
}
return graph;
}
```
接下来,我们实现一个函数来向有向图中添加边,实际上就是向邻接表中添加节点,如下所示:
```c
// 向有向图中添加边
void add_edge(Graph *graph, int start, int end) {
Node *node = (Node*)malloc(sizeof(Node));
node->data = end;
node->next = graph->vertices[start];
graph->vertices[start] = node;
graph->indegree[end]++;
}
```
然后,我们需要实现一个函数来进行拓扑排序,实现思路就是不断找出入度为0的节点,并将其输出,然后将其指向的节点的入度减1,如下所示:
```c
// 拓扑排序
void topological_sort(Graph *graph) {
int output[MAX_VERTEX_NUM]; // 存放拓扑排序结果的数组
int output_num = 0; // 拓扑排序结果的节点数目
int queue[MAX_VERTEX_NUM]; // 存放入度为0的节点的队列
int front = 0, rear = 0; // 队列的头和尾指针
for (int i = 0; i < graph->vertex_num; i++) {
if (graph->indegree[i] == 0) { // 如果入度为0,则加入队列
queue[rear++] = i;
}
}
while (front != rear) { // 不断执行,直到队列为空
int vertex = queue[front++]; // 取出队首节点
output[output_num++] = vertex; // 将节点加入拓扑排序结果数组
Node *node = graph->vertices[vertex];
while (node != NULL) { // 将该节点指向的节点的入度减1
int end = node->data;
graph->indegree[end]--;
if (graph->indegree[end] == 0) { // 如果减1后入度为0,则加入队列
queue[rear++] = end;
}
node = node->next;
}
}
if (output_num < graph->vertex_num) { // 如果拓扑排序结果的节点数目小于节点总数,则说明存在环
printf("The graph has a cycle!\n");
return;
}
printf("Topological sort result: ");
for (int i = 0; i < output_num; i++) { // 输出拓扑排序结果
printf("%d ", output[i]);
}
printf("\n");
}
```
最后,我们可以在main函数中调用上述函数来进行拓扑排序:
```c
int main() {
Graph *graph = create_graph(6);
add_edge(graph, 0, 2);
add_edge(graph, 0, 3);
add_edge(graph, 1, 3);
add_edge(graph, 1, 4);
add_edge(graph, 2, 3);
add_edge(graph, 2, 5);
add_edge(graph, 3, 5);
add_edge(graph, 4, 5);
topological_sort(graph);
return 0;
}
```
输出结果为:`Topological sort result: 0 1 2 4 3 5`,即为拓扑排序的结果。
相关推荐
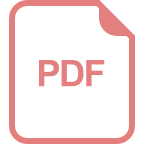
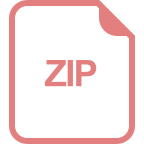














