将上述步骤以c语言的形式写成代码
时间: 2024-10-12 08:18:09 浏览: 10
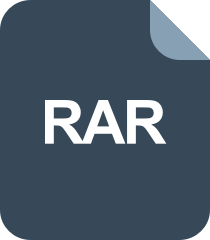
C语言写的2048
以下是使用 C 语言实现这个功能的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct ListNode {
int val;
struct ListNode* next;
} ListNode;
// 创建新节点
ListNode* createNode(int val) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (newNode) {
newNode->val = val;
newNode->next = NULL;
}
return newNode;
}
// 插入节点到链表
void insertSorted(ListNode** head, int val) {
if (*head == NULL || (*head)->val > val) {
*head = createNode(val);
} else {
ListNode* cur = *head;
while (cur->next != NULL && cur->next->val <= val) {
cur = cur->next;
}
cur->next = createNode(val);
}
}
// 计算大于 x 的元素个数
int countGreaterThan(ListNode* head, int x) {
ListNode* left = head;
ListNode* right = NULL;
int count = 0;
// 二分查找边界条件
while (left != NULL && left->val <= x) {
left = left->next;
}
// 如果找到大于 x 的节点,计数从其下一个开始
if (left != NULL) {
right = left->next;
while (right != NULL) {
if (right->val > x) {
count++;
}
right = right->next;
}
} else {
// 如果整个链表都小于等于 x,返回链长 - 1(包括 x)
count = (head == NULL) ? 0 : (head->next == NULL) ? 1 : count + 1;
}
return count;
}
int main() {
// 示例链表初始化和插入操作...
ListNode* list = createNode(1); // 假设列表已初始化
insertSorted(&list, 5);
insertSorted(&list, 2);
insertSorted(&list, 8);
int x = 4;
int result = countGreaterThan(list, x);
printf("大于 %d 的数有 %d 个\n", x, result);
return 0;
}
```
这段代码首先定义了链表节点结构和相关的插入函数,然后实现了计算大于给定值 x 的元素个数的 `countGreaterThan` 函数。`main` 函数展示了如何使用这些函数构建并查询链表。
阅读全文
相关推荐
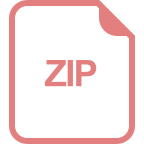
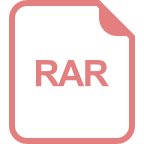
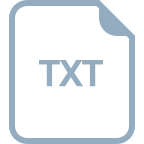
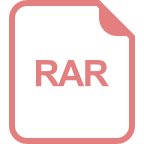
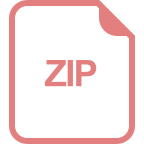
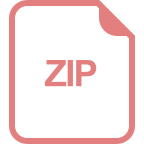
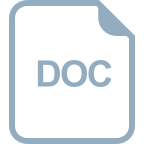
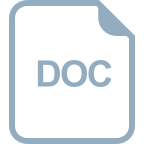
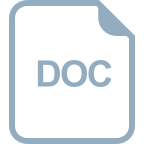
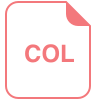
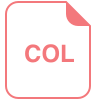
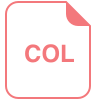
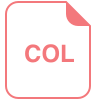
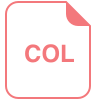
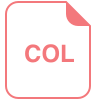
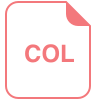


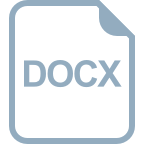