完整的surf特征点图像拼接C++代码
时间: 2024-12-25 10:35:58 浏览: 17
Surf(Speeded Up Robust Features,快速稳健特征)是一种计算机视觉中的特征检测算法,它不仅计算关键点,还同时计算关键点周围的描述符。在C++中,Surf通常是通过OpenCV库来实现的,因为OpenCV提供了对Surf和其他许多计算机视觉算法的支持。
以下是使用OpenCV C++实现SURF特征点提取和图像拼接的基本步骤,但这只是一个简化版本,实际应用中会涉及到更多的错误检查和数据处理:
```cpp
#include <opencv2/objdetect.hpp>
#include <opencv2/core.hpp>
#include <opencv2/highgui.hpp>
// 初始化SURF对象
cv::SurfFeatureDetector surfDetector;
cv::DescriptorExtractor descriptorExtractor;
// 读取图片
cv::Mat img1 = cv::imread("image1.jpg", cv::IMREAD_GRAYSCALE);
cv::Mat img2 = cv::imread("image2.jpg", cv::IMREAD_GRAYSCALE);
// 检测并匹配特征
std::vector<cv::KeyPoint> keypoints1, keypoints2;
cv::Mat descriptors1, descriptors2;
surfDetector.detect(img1, keypoints1);
surfDetector.detect(img2, keypoints2);
descriptorExtractor.compute(img1, keypoints1, descriptors1);
descriptorExtractor.compute(img2, keypoints2, descriptors2);
// 创建BFMatcher对象
cv::BFMatcher matcher(cv::NORM_L2); // 使用L2距离度量
std::vector<cv::DMatch> matches;
matcher.match(descriptors1, descriptors2, matches);
// 筛选高质量匹配
std::sort(matches.begin(), matches.end(),
[](const cv::DMatch& a, const cv::DMatch& b) { return a.distance < b.distance; });
matches.resize(std::min(matches.size(), static_cast<int>(matches.size() * 0.8)));
// 根据匹配选择对应的关键点
std::vector<cv::Point2f> points1, points2;
for (const auto& match : matches)
{
points1.push_back(keypoints1[match.queryIdx].pt);
points2.push_back(keypoints2[match.trainIdx].pt);
}
// 使用RANSAC估计变换并进行图像拼接
cv::Mat H, mask;
std::vector<cv::Point2f> srcPoints(points1), dstPoints(points2);
cv::findHomography(srcPoints, dstPoints, H, cv::RANSAC, 5, mask);
// 将两个图像根据找到的H矩阵进行融合
cv::Mat result;
cv::warpPerspective(img2, result, H, img1.size());
// 显示结果
cv::imshow("Image Stitching", result);
cv::waitKey(0);
//
阅读全文
相关推荐











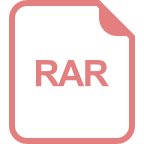


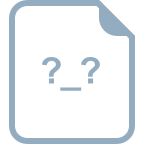



