surf特征点图像拼接C++代码
时间: 2024-12-25 08:35:19 浏览: 13
在C++中,Surf(Speeded Up Robust Features)是一种广泛用于计算机视觉的局部特征检测算法。然而,直接提供完整的代码片段会很复杂,因为这涉及到头文件、库引用以及详细的步骤。下面是一个简单的示例,展示了如何使用 Surf 特征点检测器进行图像拼接的基本框架:
```cpp
#include <opencv2/opencv.hpp>
#include <opencv2/features2d/features2d.hpp>
#include <opencv2/highgui/highgui.hpp>
// 创建 SURF对象
cv::Ptr<cv::xfeatures2d::SURF> surf = cv::xfeatures2d::SURF_create();
// 加载图片
cv::Mat img1 = cv::imread("image1.jpg");
cv::Mat img2 = cv::imread("image2.jpg");
// 检测并描述特征
std::vector<cv::KeyPoint> keypoints1, keypoints2;
cv::Mat descriptors1, descriptors2;
surf->detectAndCompute(img1, cv::noArray(), keypoints1, descriptors1);
surf->detectAndCompute(img2, cv::noArray(), keypoints2, descriptors2);
// 将特征点匹配起来(比如使用BFMatcher)
cv::BFMatcher matcher(cv::NORM_L2); // 使用L2距离衡量
std::vector<cv::DMatch> matches;
matcher.match(descriptors1, descriptors2, matches);
// 筛选高质量的匹配(例如,只保留前N个最好的)
std::sort(matches.begin(), matches.end(), [](const cv::DMatch& a, const cv::DMatch& b) { return a.distance < b.distance; });
matches.resize(40); // 或者选择其他适合的数目
// 根据匹配找到对应像素,并尝试图像拼接
std::vector<cv::Point2f> points1, points2;
for (const auto& match : matches)
{
points1.push_back(keypoints1[match.queryIdx].pt);
points2.push_back(keypoints2[match.trainIdx].pt);
}
cv::Rect rect = findHomography(points1, points2).boundingRect(); // 找到变换矩阵,然后获取拼接区域
cv::Mat result;
cv::warpAffine(img2, result, rect, img1.size());
// 显示结果
cv::imshow("Image Stitching", result);
cv::waitKey(0);
```
请注意,这只是一个基本示例,实际应用中可能需要更复杂的错误处理和数据预处理步骤。此外,`findHomography`函数在这里假设你知道如何计算单应性矩阵,通常需要使用 RANSAC 算法等优化手段。
阅读全文
相关推荐

















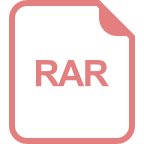
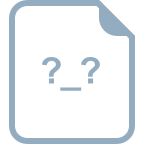