sift+FLANN+ransan+加权融合算法的代码
时间: 2023-09-23 12:11:33 浏览: 60
这里提供一个基于OpenCV实现的sift+FLANN+ransac+加权融合算法的代码,代码中使用了两幅图像进行拼接。需要注意的是,这段代码仅供参考,具体实现需要根据实际情况进行调整和改进。
```python
import cv2
import numpy as np
# 读入两幅图片
img1 = cv2.imread('img1.jpg')
img2 = cv2.imread('img2.jpg')
# 获取图片大小
rows1, cols1 = img1.shape[:2]
rows2, cols2 = img2.shape[:2]
# 使用sift算法提取特征点和特征描述子
sift = cv2.xfeatures2d.SIFT_create()
keypoints1, descriptors1 = sift.detectAndCompute(img1, None)
keypoints2, descriptors2 = sift.detectAndCompute(img2, None)
# 使用FLANN算法进行特征点匹配
FLANN_INDEX_KDTREE = 0
index_params = dict(algorithm=FLANN_INDEX_KDTREE, trees=5)
search_params = dict(checks=50)
flann = cv2.FlannBasedMatcher(index_params, search_params)
matches = flann.knnMatch(descriptors1, descriptors2, k=2)
# 进行ransac过滤
good_matches = []
for m, n in matches:
if m.distance < 0.7 * n.distance:
good_matches.append(m)
src_pts = np.float32([keypoints1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([keypoints2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 计算拼接后的图像大小
h, w = img1.shape[:2]
pts = np.float32([[0, 0], [0, h - 1], [w - 1, h - 1], [w - 1, 0]]).reshape(-1, 1, 2)
dst = cv2.perspectiveTransform(pts, M)
dst += (cols1, 0)
# 进行图像拼接
result = np.zeros((max(rows1, rows2), cols1 + cols2, 3), dtype=np.uint8)
result[:rows1, :cols1, :] = img1
result[:rows2, cols1:, :] = img2
# 加权融合
for i in range(dst.shape[0]):
x, y = dst[i][0]
x, y = int(x), int(y)
if 0 <= x < cols1 + cols2 and 0 <= y < max(rows1, rows2):
if x < cols1:
result[y, x, :] = img1[y, x, :]
elif x >= cols1:
result[y, x, :] = img2[y, x - cols1, :]
else:
result[y, x, :] = (img1[y, x, :] + img2[y, x - cols1, :]) / 2
# 显示拼接后的图像
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
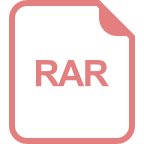














