python拟合函数图像
时间: 2023-07-21 09:00:23 浏览: 91
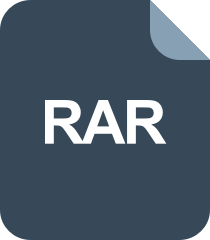
3d_fitting函数图像拟合
你可以使用Python中的matplotlib库来拟合函数图像。下面是一个简单的例子,展示了如何使用numpy和matplotlib来拟合并绘制函数的图像:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义函数
def my_function(x):
return np.sin(x)
# 生成x轴的数据
x = np.linspace(0, 2*np.pi, 100)
# 计算对应的y轴数据
y = my_function(x)
# 绘制函数图像
plt.plot(x, y)
plt.xlabel('x')
plt.ylabel('y')
plt.title('Function Plot')
plt.grid(True)
plt.show()
```
在这个例子中,我们定义了一个简单的函数`my_function(x)`,然后使用`np.linspace()`函数生成了一组等间距的x轴数据。接下来,我们通过调用`my_function(x)`计算出对应的y轴数据。最后,使用`plt.plot()`函数绘制函数的图像,并通过其他函数设置了图例、坐标轴标签和标题等。
你可以根据自己的需求修改函数和x轴的范围,来拟合并绘制其他函数的图像。希望这可以帮助到你!如果有任何进一步的问题,请随时提问。
阅读全文
相关推荐
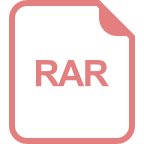




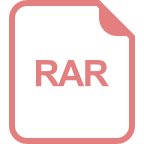
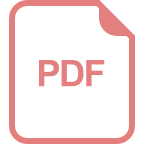









