flowlayout如何打印文本框
时间: 2023-07-09 21:33:00 浏览: 52
FlowLayout 是 Java 中的一种布局管理器,用于在容器中排列组件。它并不直接涉及文本框的打印,但可以用于布局包含文本框的容器。
如果您想打印文本框的内容,可以使用 Java 中的打印 API。以下是一个简单的示例,演示如何使用 JTextArea 和 FlowLayout 来打印文本框的内容:
```java
import java.awt.*;
import java.awt.print.*;
import javax.swing.*;
public class PrintText extends JFrame implements Printable {
private JTextArea textArea;
public PrintText() {
setTitle("Print Text");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create a JTextArea to hold the text to be printed
textArea = new JTextArea();
textArea.setText("Hello, world!");
// Add the JTextArea to a JPanel with a FlowLayout
JPanel panel = new JPanel(new FlowLayout());
panel.add(textArea);
add(panel);
// Create a Print button to trigger printing
JButton printButton = new JButton("Print");
printButton.addActionListener(e -> {
// Call the print() method to print the JTextArea contents
PrinterJob job = PrinterJob.getPrinterJob();
job.setPrintable(this);
if (job.printDialog()) {
try {
job.print();
} catch (PrinterException ex) {
ex.printStackTrace();
}
}
});
panel.add(printButton);
}
// Implement the Printable interface to provide the content to be printed
public int print(Graphics g, PageFormat format, int pageIndex)
throws PrinterException {
if (pageIndex > 0) {
return NO_SUCH_PAGE;
}
// Get the font size and line height of the JTextArea
Font font = textArea.getFont();
FontMetrics metrics = textArea.getFontMetrics(font);
int lineHeight = metrics.getHeight();
// Set the starting position for the text to be printed
int x = (int) format.getImageableX();
int y = (int) format.getImageableY() + lineHeight;
// Split the JTextArea contents into lines and print each line
String[] lines = textArea.getText().split("\\n");
for (String line : lines) {
g.drawString(line, x, y);
y += lineHeight;
}
return PAGE_EXISTS;
}
public static void main(String[] args) {
new PrintText().setVisible(true);
}
}
```
这个例子创建了一个包含一个 JTextArea 和一个打印按钮的窗口。当用户点击打印按钮时,程序会调用 print() 方法,将 JTextArea 中的内容打印出来。在 print() 方法中,我们获取了 JTextArea 的字体大小和行高,然后将文本分成多行逐行打印到页面上。
相关推荐
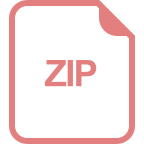
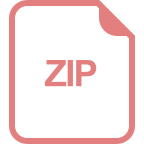
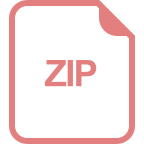














