ffmpeg rtsp调用av_read_frame读取实时视频流返回AVERROR_EOF
时间: 2023-06-15 21:07:11 浏览: 103
AVERROR_EOF表示已经到达了流的末尾,无法再读取更多的数据。如果你使用av_read_frame读取实时视频流返回AVERROR_EOF,可能是因为服务器端断开了连接或者出现了其他异常情况导致数据流中断。你可以尝试重新连接服务器或者检查网络连接是否正常。另外,你也可以考虑使用类似于FFmpeg的推流工具推送本地视频流到服务器端,这样可以避免一些网络连接问题。
相关问题
ffmpeg rtsp视频流解码的时候如何抽帧 c++
在 C++ 中使用 FFmpeg 抽取 RTSP 视频流的帧,可以按照以下步骤进行:
1. 初始化 FFmpeg 库
```c++
#include <iostream>
extern "C" {
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/imgutils.h>
#include <libswscale/swscale.h>
}
int main() {
// 注册 FFmpeg 库中的所有可用的文件格式和编解码器
av_register_all();
return 0;
}
```
2. 打开 RTSP 视频流
```c++
AVFormatContext* format_context = nullptr;
AVCodecContext* codec_context = nullptr;
AVCodec* codec = nullptr;
// 打开 RTSP 视频流并读取视频流信息
if (avformat_open_input(&format_context, "rtsp://xxx.xxx.xxx.xxx:xxxx/xxx", nullptr, nullptr) != 0) {
std::cout << "无法打开 RTSP 视频流" << std::endl;
return -1;
}
// 获取视频流信息
if (avformat_find_stream_info(format_context, nullptr) < 0) {
std::cout << "无法获取视频流信息" << std::endl;
return -1;
}
// 查找视频流
int video_stream_index = -1;
for (int i = 0; i < format_context->nb_streams; i++) {
if (format_context->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
video_stream_index = i;
break;
}
}
if (video_stream_index == -1) {
std::cout << "无法找到视频流" << std::endl;
return -1;
}
// 获取视频流编解码器
codec_context = avcodec_alloc_context3(nullptr);
if (!codec_context) {
std::cout << "无法分配视频流编解码器上下文" << std::endl;
return -1;
}
avcodec_parameters_to_context(codec_context, format_context->streams[video_stream_index]->codecpar);
codec = avcodec_find_decoder(codec_context->codec_id);
if (!codec) {
std::cout << "无法找到视频流编解码器" << std::endl;
return -1;
}
// 打开视频流编解码器
if (avcodec_open2(codec_context, codec, nullptr) < 0) {
std::cout << "无法打开视频流编解码器" << std::endl;
return -1;
}
```
3. 循环读取视频流帧
```c++
AVFrame* frame = nullptr;
AVPacket packet;
int frame_count = 0;
while (av_read_frame(format_context, &packet) >= 0) {
// 判断是否为视频流包
if (packet.stream_index == video_stream_index) {
// 解码视频流帧
frame = av_frame_alloc();
int ret = avcodec_send_packet(codec_context, &packet);
if (ret < 0) {
std::cout << "无法向视频流编解码器发送数据" << std::endl;
break;
}
while (ret >= 0) {
ret = avcodec_receive_frame(codec_context, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
}
else if (ret < 0) {
std::cout << "无法解码视频流帧" << std::endl;
goto end;
}
// 处理视频流帧
std::cout << "第 " << ++frame_count << " 帧" << std::endl;
// 释放帧数据
av_frame_unref(frame);
}
}
// 释放视频流包
av_packet_unref(&packet);
}
end:
// 释放资源
avcodec_free_context(&codec_context);
avformat_close_input(&format_context);
```
以上是抽取 RTSP 视频流帧的基本步骤,具体实现还需要根据实际需求进行调整。
ffmpeg 获取 rtsp 视频流 c++ 代码实现
下面是一个简单的 C++ 代码实现,使用 FFmpeg 库获取 RTSP 视频流:
```
extern "C"
{
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/imgutils.h>
#include <libswscale/swscale.h>
#include <libavutil/opt.h>
}
#include <iostream>
using namespace std;
int main()
{
int ret;
// 初始化 FFmpeg 库
av_register_all();
// 打开 RTSP 链接
AVFormatContext* fmt_ctx = NULL;
ret = avformat_open_input(&fmt_ctx, "rtsp://xxx.xxx.xxx.xxx:xxxx/xxx", NULL, NULL);
if (ret < 0)
{
cerr << "Could not open RTSP input: " << av_err2str(ret) << endl;
return -1;
}
// 查找流信息
ret = avformat_find_stream_info(fmt_ctx, NULL);
if (ret < 0)
{
cerr << "Could not find RTSP stream information: " << av_err2str(ret) << endl;
avformat_close_input(&fmt_ctx);
return -1;
}
// 找到视频流
AVCodec* video_codec = NULL;
int video_stream_index = av_find_best_stream(fmt_ctx, AVMEDIA_TYPE_VIDEO, -1, -1, &video_codec, 0);
if (video_stream_index < 0)
{
cerr << "Could not find RTSP video stream: " << av_err2str(video_stream_index) << endl;
avformat_close_input(&fmt_ctx);
return -1;
}
// 打开视频编解码器
AVCodecContext* codec_ctx = avcodec_alloc_context3(video_codec);
if (!codec_ctx)
{
cerr << "Could not allocate video codec context" << endl;
avformat_close_input(&fmt_ctx);
return -1;
}
ret = avcodec_parameters_to_context(codec_ctx, fmt_ctx->streams[video_stream_index]->codecpar);
if (ret < 0)
{
cerr << "Could not copy codec parameters to context: " << av_err2str(ret) << endl;
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
return -1;
}
ret = avcodec_open2(codec_ctx, video_codec, NULL);
if (ret < 0)
{
cerr << "Could not open video codec: " << av_err2str(ret) << endl;
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
return -1;
}
// 准备解码视频帧
AVFrame* frame = av_frame_alloc();
if (!frame)
{
cerr << "Could not allocate video frame" << endl;
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
return -1;
}
AVPacket packet;
av_init_packet(&packet);
// 初始化视频转换器
SwsContext* sws_ctx = sws_getContext(codec_ctx->width, codec_ctx->height, codec_ctx->pix_fmt,
codec_ctx->width, codec_ctx->height, AV_PIX_FMT_RGB24,
0, NULL, NULL, NULL);
if (!sws_ctx)
{
cerr << "Could not initialize video converter" << endl;
av_frame_free(&frame);
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
return -1;
}
// 循环读取视频帧
int frame_count = 0;
while (av_read_frame(fmt_ctx, &packet) >= 0)
{
// 只处理视频帧
if (packet.stream_index == video_stream_index)
{
// 解码视频帧
ret = avcodec_send_packet(codec_ctx, &packet);
if (ret < 0)
{
cerr << "Error sending a packet for decoding: " << av_err2str(ret) << endl;
break;
}
while (ret >= 0)
{
ret = avcodec_receive_frame(codec_ctx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
{
break;
}
else if (ret < 0)
{
cerr << "Error during decoding: " << av_err2str(ret) << endl;
break;
}
// 转换视频帧格式
uint8_t* data[1] = { (uint8_t*)frame->data[0] };
int linesize[1] = { frame->linesize[0] };
sws_scale(sws_ctx, data, linesize, 0, codec_ctx->height, frame->data, frame->linesize);
// 在这里可以对视频帧进行处理
frame_count++;
}
}
av_packet_unref(&packet);
}
// 释放资源
sws_freeContext(sws_ctx);
av_frame_free(&frame);
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
cout << "Processed " << frame_count << " video frames" << endl;
return 0;
}
```
请注意,此代码仅用于演示目的,实际使用需要进行适当的错误处理和资源释放。
相关推荐
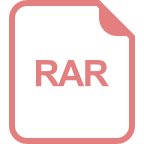












