使用Java代码分析人羊狼菜过河问题
时间: 2023-09-20 16:11:39 浏览: 130
人羊狼菜过河问题是一个经典的逻辑推理问题,可以使用Java代码进行分析和解决。以下是一个简单的Java代码示例,通过深度优先搜索(DFS)算法来解决该问题。
```java
import java.util.*;
public class RiverCrossingProblem {
// 定义人羊狼菜过河问题的初始状态
private static final int LEFT = 0;
private static final int RIGHT = 1;
private static final int[] INIT_STATE = {LEFT, LEFT, LEFT, LEFT};
// 定义人羊狼菜过河问题的目标状态
private static final int[] GOAL_STATE = {RIGHT, RIGHT, RIGHT, RIGHT};
// 定义人、羊、狼、菜的编号
private static final int PERSON = 0;
private static final int SHEEP = 1;
private static final int WOLF = 2;
private static final int VEGETABLE = 3;
// 定义人羊狼菜过河问题的状态
private static class State {
int[] items;
int boat;
State parent;
public State(int[] items, int boat, State parent) {
this.items = items;
this.boat = boat;
this.parent = parent;
}
public boolean isGoal() {
return Arrays.equals(items, GOAL_STATE);
}
public List<State> getNextStates() {
List<State> nextStates = new ArrayList<>();
for (int i = 0; i < items.length; i++) {
if (items[i] != boat) continue;
int[] nextItems = items.clone();
nextItems[i] = 1 - nextItems[i];
if (isValidState(nextItems)) {
nextStates.add(new State(nextItems, 1 - boat, this));
}
for (int j = i + 1; j < items.length; j++) {
if (items[j] != boat) continue;
int[] nextItems2 = nextItems.clone();
nextItems2[j] = 1 - nextItems2[j];
if (isValidState(nextItems2)) {
nextStates.add(new State(nextItems2, 1 - boat, this));
}
}
}
return nextStates;
}
private boolean isValidState(int[] items) {
if (items[SHEEP] == items[WOLF] && items[PERSON] != items[SHEEP]) {
return false;
}
if (items[VEGETABLE] == items[SHEEP] && items[PERSON] != items[VEGETABLE]) {
return false;
}
return true;
}
}
// 使用深度优先搜索算法来解决人羊狼菜过河问题
public static void solve() {
Stack<State> stack = new Stack<>();
Set<String> visited = new HashSet<>();
State initState = new State(INIT_STATE, LEFT, null);
stack.push(initState);
visited.add(Arrays.toString(initState.items));
while (!stack.isEmpty()) {
State currentState = stack.pop();
if (currentState.isGoal()) {
printPath(currentState);
return;
}
for (State nextState : currentState.getNextStates()) {
if (!visited.contains(Arrays.toString(nextState.items))) {
stack.push(nextState);
visited.add(Arrays.toString(nextState.items));
}
}
}
System.out.println("No solution found!");
}
// 打印求解路径
private static void printPath(State state) {
List<String> path = new ArrayList<>();
while (state != null) {
path.add(Arrays.toString(state.items));
state = state.parent;
}
Collections.reverse(path);
for (String s : path) {
System.out.println(s);
}
}
public static void main(String[] args) {
solve();
}
}
```
在上述代码中,我们首先定义了人羊狼菜过河问题的初始状态和目标状态,以及人、羊、狼、菜的编号。然后,我们定义了一个`State`类来表示人羊狼菜过河问题的状态,包括当前状态下的物品位置、船的位置和父状态。`State`类还包括了判断当前状态是否为目标状态、获取下一步状态的方法以及判断状态是否合法的方法。
接下来,我们使用深度优先搜索算法来解决人羊狼菜过河问题。我们首先定义了一个栈和一个HashSet,用于存储当前搜索路径和已经访问过的状态。然后,我们从初始状态开始搜索,每次从栈中弹出一个状态,并查找该状态下所有可能的下一步状态。如果某个下一步状态是合法的且之前没有访问过,那么将其压入栈中,并将其添加到已访问状态的HashSet中。如果找到了目标状态,则打印求解路径;否则,输出“No solution found!”。最后,在`main`方法中调用`solve`方法来求解人羊狼菜过河问题。
需要注意的是,上述代码只是一个简单的示例,还有许多可以改进的地方,例如使用广度优先搜索算法、优化搜索过程等等。
阅读全文
相关推荐





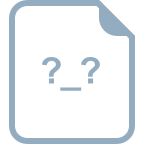
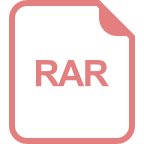






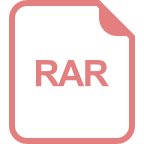