tensorflow tsne可视化
时间: 2023-10-06 21:04:12 浏览: 177
TensorFlow提供了一种用于可视化高维数据的降维技术,称为t-SNE(t-Distributed Stochastic Neighbor Embedding)。t-SNE可以将高维数据映射到一个二维或三维空间中,以便更容易进行可视化和理解。
要在TensorFlow中使用t-SNE进行可视化,你需要遵循以下步骤:
1. 准备数据:将你的高维数据准备好,并确保数据格式适合输入到TensorFlow中。
2. 定义模型:在TensorFlow中,你需要定义一个t-SNE模型。你可以使用TensorFlow的高级API(如Keras)来定义和训练模型。
3. 训练模型:使用准备好的数据来训练t-SNE模型。在训练过程中,模型会学习如何将高维数据映射到低维空间。
4. 可视化数据:使用训练好的t-SNE模型,将高维数据映射到二维或三维空间,并将其可视化。你可以使用matplotlib等库来呈现可视化结果。
这是一个基本的框架,你可以根据自己的需求进行进一步的调整和改进。请注意,t-SNE是一种计算密集型算法,处理大规模数据可能需要更多的计算资源和时间。
希望这些信息对你有所帮助!如果你有更多关于TensorFlow或t-SNE的问题,可以继续提问。
相关问题
tensorflow tsne分裂可视化
您好!对于TensorFlow中的t-SNE分类可视化,您可以按照以下步骤进行操作:
1. 导入必要的库和模块:
```python
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
from sklearn.manifold import TSNE
```
2. 准备数据:
假设您有一个特征矩阵`features`和对应的标签`labels`,您可以将其转换为NumPy数组:
```python
features = np.array(features)
labels = np.array(labels)
```
3. 运行t-SNE算法:
```python
tsne = TSNE(n_components=2, random_state=0)
embeddings = tsne.fit_transform(features)
```
4. 可视化结果:
```python
plt.scatter(embeddings[:, 0], embeddings[:, 1], c=labels)
plt.show()
```
上述代码将生成一个散点图,其中x轴和y轴表示t-SNE算法生成的两个维度,颜色表示实际的类别标签。
请注意,上述代码仅提供了一个简单的示例,您可能需要根据您的具体数据进行适当的调整和修改。另外,确保已安装所需的库和模块,例如matplotlib和scikit-learn。
希望这可以帮助到您!如果您有任何其他问题,请随时提问。
卷积神经网络tsne可视化代码
以下是使用Python和TensorFlow的卷积神经网络TSNE可视化代码示例:
```python
import numpy as np
import tensorflow as tf
import matplotlib.pyplot as plt
from sklearn.manifold import TSNE
# 加载数据集
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
# 将像素值标准化为0到1之间的实数
x_train = x_train.astype(np.float32) / 255.
x_test = x_test.astype(np.float32) / 255.
# 调整输入形状以适应模型
x_train = np.expand_dims(x_train, axis=-1)
x_test = np.expand_dims(x_test, axis=-1)
# 定义卷积神经网络模型
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3,3), activation='relu', input_shape=(28,28,1)),
tf.keras.layers.MaxPooling2D((2,2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(10, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=5, validation_data=(x_test, y_test))
# 提取卷积层的输出
layer_output = model.layers[0].output
feature_model = tf.keras.models.Model(inputs=model.inputs, outputs=layer_output)
features = feature_model.predict(x_test)
# 使用TSNE进行降维
tsne = TSNE(n_components=2, perplexity=30, n_iter=1000, random_state=0)
tsne_features = tsne.fit_transform(features.reshape(features.shape[0], -1))
# 可视化TSNE降维后的特征
plt.scatter(tsne_features[:,0], tsne_features[:,1], c=y_test, cmap='jet')
plt.show()
```
在这个示例中,我们使用MNIST数据集训练了一个简单的卷积神经网络模型,并提取了卷积层的输出。然后,我们使用TSNE将这些特征降到2维,并用散点图进行可视化。每个数据点的颜色表示它属于哪个数字类别。结果是一个紧密聚集的散点图,显示了数字类别之间的分离。
阅读全文
相关推荐
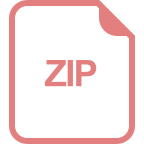
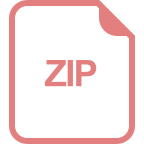
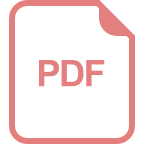
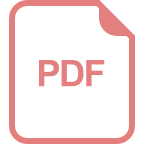


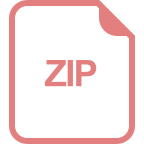
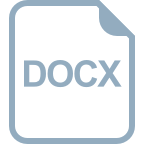
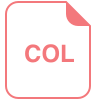
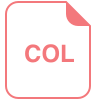
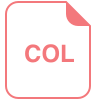




