import matplotlib.ticker as mtick
时间: 2024-01-06 18:06:07 浏览: 38
`matplotlib.ticker`是Matplotlib库中用于绘制坐标轴刻度线和标签的工具包。`mtick`模块提供了一些常用的刻度线格式化器和定位器。比如可以使用`PercentFormatter`来将小数转换为百分数形式,也可以使用`MultipleLocator`来设置坐标轴刻度的间隔。
以下是一个简单例子,演示如何使用`mtick`模块来设置y轴刻度为百分数形式:
``` python
import matplotlib.pyplot as plt
import matplotlib.ticker as mtick
import numpy as np
x = np.linspace(0, 1, 11)
y = np.random.rand(11)
fig, ax = plt.subplots()
ax.plot(x, y)
# 设置y轴刻度为百分数形式
fmt = '%.0f%%' # 将小数转换为百分数,保留0位小数
yticks = mtick.FormatStrFormatter(fmt)
ax.yaxis.set_major_formatter(yticks)
plt.show()
```
运行以上代码,将会得到一张图像,y轴刻度显示为百分数形式。
相关问题
import matplotlib.ticker as ticker
Matplotlib.ticker是Matplotlib库中的一个模块,用于在图表中设置刻度定位器和格式化器。它可以帮助我们更好地控制坐标轴的显示效果。
常见的刻度定位器和格式化器包括:
1. FixedLocator:指定固定的刻度位置;
2. MultipleLocator:指定刻度间隔;
3. AutoLocator:自动选择刻度间隔;
4. NullLocator:不显示刻度;
5. FormatStrFormatter:用指定的格式化字符串格式化刻度值;
6. ScalarFormatter:用科学计数法格式化刻度值。
下面是一个简单示例,展示如何使用刻度定位器和格式化器:
```python
import matplotlib.pyplot as plt
import matplotlib.ticker as ticker
# 生成数据
x = [1, 2, 3, 4, 5]
y = [10, 20, 15, 25, 30]
# 绘制折线图
plt.plot(x, y)
# 设置x轴刻度定位器和格式化器
x_locator = ticker.MultipleLocator(1) # 设置间隔为1
x_formatter = ticker.FormatStrFormatter('%d') # 格式化为整数
plt.gca().xaxis.set_major_locator(x_locator)
plt.gca().xaxis.set_major_formatter(x_formatter)
# 设置y轴刻度定位器和格式化器
y_locator = ticker.MultipleLocator(5) # 设置间隔为5
y_formatter = ticker.ScalarFormatter() # 使用科学计数法
plt.gca().yaxis.set_major_locator(y_locator)
plt.gca().yaxis.set_major_formatter(y_formatter)
# 显示图形
plt.show()
```
运行代码后,会得到一个折线图,其中x轴的刻度间隔为1,格式为整数;y轴的刻度间隔为5,使用科学计数法显示。
import matplotlib.plot as plt
`import matplotlib.plot as plt` 是错误的导入语句,正确的导入语句应该是 `import matplotlib.pyplot as plt`。这个语句的作用是导入 `matplotlib.pyplot` 模块,并将其重命名为 `plt`,以便在代码中更方便地使用。
`matplotlib.pyplot` 是 Matplotlib 库中的一个子模块,它提供了一系列用于绘制图形的函数。通过导入 `matplotlib.pyplot`,我们可以使用其中的函数来创建各种类型的图形,如折线图、散点图、柱状图等。
以下是一个简单的例子,演示了如何使用 `matplotlib.pyplot` 绘制折线图:
```python
import matplotlib.pyplot as plt
# 创建 x 和 y 数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 绘制折线图
plt.plot(x, y)
# 添加标题和标签
plt.title("Line Plot")
plt.xlabel("X")
plt.ylabel("Y")
# 显示图形
plt.show()
```
这段代码首先导入了 `matplotlib.pyplot` 模块,并将其重命名为 `plt`。然后,创建了 x 和 y 数据,分别表示横轴和纵轴的值。接下来,使用 `plt.plot()` 函数绘制了折线图。最后,使用 `plt.title()`、`plt.xlabel()` 和 `plt.ylabel()` 函数添加了标题和标签,并使用 `plt.show()` 函数显示了图形。
相关推荐
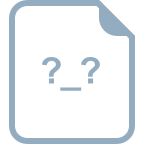
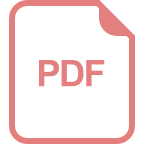









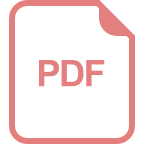
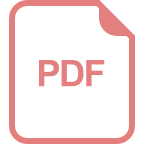
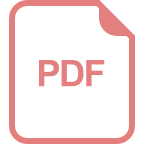
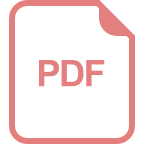