仔细阅读教材P126Person类按姓名比较对象大小的例子,为前面作业中的Complex类实现Comparable<T>接口,提供按模比较复数大小的方法。
时间: 2023-07-15 20:10:53 浏览: 118
好的,这里是实现按模比较复数大小的代码:
```java
public class Complex implements Comparable<Complex> {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public double getReal() {
return real;
}
public void setReal(double real) {
this.real = real;
}
public double getImag() {
return imag;
}
public void setImag(double imag) {
this.imag = imag;
}
public double getMod() {
return Math.sqrt(real * real + imag * imag);
}
@Override
public int compareTo(Complex o) {
if (this.getMod() < o.getMod()) {
return -1;
} else if (this.getMod() > o.getMod()) {
return 1;
} else {
return 0;
}
}
}
```
在该代码中,我们实现了 `Comparable<Complex>` 接口,并重写了 `compareTo` 方法。该方法比较两个复数对象的模大小,如果调用当前对象的 `getMod()` 方法得到的模比参数对象的模小,则返回负数;如果调用当前对象的 `getMod()` 方法得到的模比参数对象的模大,则返回正数;如果两者模相等,则返回0。这符合 `Comparable<T>` 接口的约定。
现在,我们可以通过调用 `Collections.sort()` 方法来对复数列表进行排序,排序时会自动调用 `compareTo` 方法,按照模大小进行排序。例如:
```java
List<Complex> complexList = new ArrayList<>();
complexList.add(new Complex(3, 4));
complexList.add(new Complex(1, 1));
complexList.add(new Complex(2, -1));
Collections.sort(complexList);
System.out.println(complexList);
// 输出:[Complex [real=2.0, imag=-1.0], Complex [real=1.0, imag=1.0], Complex [real=3.0, imag=4.0]]
```
可以看到,排序后,复数列表中的元素按照模大小从小到大排列。
阅读全文
相关推荐
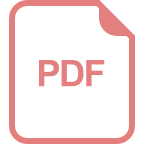
















