用Python写一个贪吃蛇游戏代码
时间: 2024-03-28 22:17:23 浏览: 16
好的,我可以为您提供一个Python写的贪吃蛇游戏代码。请注意,这是基于Python 3版本的代码。以下是代码:
```python
import tkinter as tk
import random
class Snake(tk.Canvas):
def __init__(self):
super().__init__(width=600, height=620, background="black", highlightthickness=0)
self.active = True
self.snake_positions = [(100, 100), (80, 100), (60, 100)]
self.food_position = self.set_new_food_position()
self.score = 0
self.direction = "Right"
self.bind_all("<Key>", self.on_key_press)
self.score_text = self.create_text(45, 12, text=f"Score: {self.score}",
fill="#fff", font=("TkDefaultFont", 14))
self.focus_set()
self.change_direction()
self.mainloop()
def on_key_press(self, event):
new_direction = event.keysym
all_directions = ("Up", "Down", "Left", "Right")
opposites = ({"Up", "Down"}, {"Left", "Right"})
if (new_direction in all_directions) and ({new_direction, self.direction} not in opposites):
self.direction = new_direction
def move_snake(self):
head_x, head_y = self.snake_positions[0]
if self.direction == "Left":
new_head_position = (head_x-20, head_y)
elif self.direction == "Right":
new_head_position = (head_x+20, head_y)
elif self.direction == "Up":
new_head_position = (head_x, head_y-20)
elif self.direction == "Down":
new_head_position = (head_x, head_y+20)
self.snake_positions = [new_head_position] + self.snake_positions[:-1]
for segment, position in zip (self.find_withtag("snake"), self.snake_positions):
self.coords(segment, position)
def change_direction(self):
if self.active:
self.move_snake()
self.check_collisions()
self.check_food_collision()
self.after(100, self.change_direction)
def set_new_food_position(self):
while True:
x_position = random.randint(1, 29) * 20
y_position = random.randint(3, 30) * 20
food_position = (x_position, y_position)
if food_position not in self.snake_positions:
return food_position
def check_food_collision(self):
if self.snake_positions[0] == self.food_position:
self.snake_positions.append(self.snake_positions[-1])
self.create_image(*self.snake_positions[-1], image=self.snake_body, tag="snake")
self.food_position = self.set_new_food_position()
self.score += 10
self.itemconfig(self.score_text, text=f"Score: {self.score}", fill="#fff")
def check_collisions(self):
head_x, head_y = self.snake_positions[0]
body = self.snake_positions[1:]
crosses = [position for position in body if position == (head_x, head_y)]
if (head_x < 0) or (head_x > 580) or (head_y < 20) or (head_y > 600) or crosses:
self.game_over()
def game_over(self):
self.active = False
self.delete(tk.ALL)
self.create_text(self.winfo_width()/2, self.winfo_height()/2,
text=f"Game Over!\nFinal Score: {self.score}", fill="#fff", font=("TkDefaultFont", 14))
if __name__ == "__main__":
root = tk.Tk()
root.title("Snake")
snake_game = Snake()
```
这段代码将创建一个窗口,其中包含一个可移动的贪吃蛇和一个随机放置的食物。当贪吃蛇吃掉食物时,它会变得更长,并增加玩家的分数。如果贪吃蛇碰到窗口的边缘或自己的身体,则游戏结束。
相关推荐
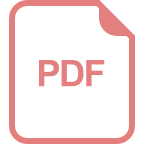












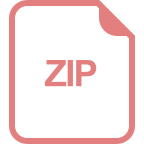
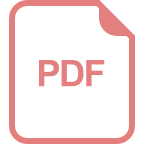