帮我用python写一个贪吃蛇的代码
时间: 2024-09-23 20:13:36 浏览: 46
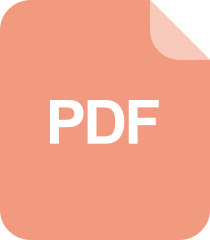
使用Python写一个贪吃蛇游戏实例代码
当然,这是一个基本的Python贪吃蛇游戏的框架。首先,我们需要导入必要的模块,并定义一些常量和函数。游戏的核心是蛇的身体移动、食物生成以及碰撞检测。以下是简化的代码示例:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置窗口大小和标题
screen_width = 400
screen_height = 400
window = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("贪吃蛇")
snake_speed = 5
snake_block = 10
food_spawn_radius = 15
# 蛇的位置和方向
snake = [(screen_width / 2, screen_height / 2), (0, snake_block)]
direction = 'right'
def move_snake():
global snake
if direction == 'up':
snake[0] = (snake[0][0], snake[0][1] - snake_block)
elif direction == 'down':
snake[0] = (snake[0][0], snake[0][1] + snake_block)
elif direction == 'left':
snake[0] = (snake[0][0] - snake_block, snake[0][1])
else:
snake[0] = (snake[0][0] + snake_block, snake[0][1])
def generate_food():
while True:
x = random.randint(0, screen_width // snake_block) * snake_block
y = random.randint(0, screen_height // snake_block) * snake_block
if (x, y) not in snake:
return (x, y)
food = generate_food()
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP and direction != 'down':
direction = 'up'
elif event.key == pygame.K_DOWN and direction != 'up':
direction = 'down'
elif event.key == pygame.K_LEFT and direction != 'right':
direction = 'left'
elif event.key == pygame.K_RIGHT and direction != 'left':
direction = 'right'
# 移动蛇并检测碰撞
move_snake()
if snake[0] in food or snake[0] in snake[1:]:
print("Game Over!")
running = False
else:
window.fill((0, 0, 0))
# 绘制元素
pygame.draw.rect(window, (255, 0, 0), pygame.Rect(food[0], food[1], snake_block, snake_block))
for body_part in snake[:-1]:
pygame.draw.rect(window, (0, 255, 0), pygame.Rect(body_part[0], body_part[1], snake_block, snake_block))
# 更新屏幕
pygame.display.update()
pygame.time.delay(snake_speed)
pygame.quit()
```
你可以运行这个代码开始玩一个最基础的贪吃蛇游戏。注意这只是一个起点,实际游戏中你可以添加更多的功能,比如增加分值、蛇的增长、蛇头吃到身体等。
阅读全文
相关推荐
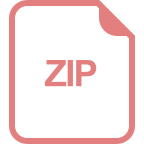
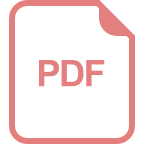








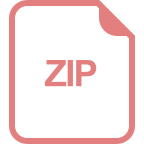
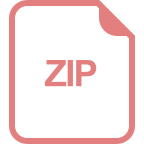
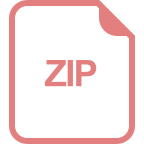