msp430 配置 lora 模块的代码
时间: 2024-06-08 08:09:19 浏览: 19
以下是一个基于 MSP430 单片机的 LoRa 模块配置示例代码,使用的是 Semtech 公司的 SX1276 模块:
```c
#include <msp430.h>
#include <stdint.h>
#define RESET_PIN BIT0
#define DIO0_PIN BIT1
#define SPI_CLK_PIN BIT5
#define SPI_MISO_PIN BIT6
#define SPI_MOSI_PIN BIT7
#define SEL_PIN BIT4
void SPI_Write(uint8_t addr, uint8_t data)
{
P1OUT &= ~SEL_PIN; // Set SEL pin to low to select the slave
UCB0TXBUF = addr | 0x80; // Send the address with MSB set to 1 to indicate a write operation
while(!(UCB0IFG & UCTXIFG)); // Wait until the transmission is complete
UCB0TXBUF = data; // Send the data byte
while(!(UCB0IFG & UCTXIFG)); // Wait until the transmission is complete
P1OUT |= SEL_PIN; // Set SEL pin to high to deselect the slave
}
uint8_t SPI_Read(uint8_t addr)
{
uint8_t data;
P1OUT &= ~SEL_PIN; // Set SEL pin to low to select the slave
UCB0TXBUF = addr & 0x7F; // Send the address with MSB set to 0 to indicate a read operation
while(!(UCB0IFG & UCTXIFG)); // Wait until the transmission is complete
UCB0TXBUF = 0; // Send a dummy byte to receive the data byte
while(!(UCB0IFG & UCRXIFG)); // Wait until the reception is complete
data = UCB0RXBUF; // Read the received data byte
P1OUT |= SEL_PIN; // Set SEL pin to high to deselect the slave
return data;
}
void LoRa_Reset()
{
P1OUT &= ~RESET_PIN; // Set RESET pin to low to reset the module
__delay_cycles(10000); // Wait for 10ms
P1OUT |= RESET_PIN; // Set RESET pin to high to release the module from reset
__delay_cycles(10000); // Wait for 10ms
}
void LoRa_Init()
{
// Configure the SPI pins as outputs
P1DIR |= SEL_PIN | SPI_CLK_PIN | SPI_MOSI_PIN;
P1DIR &= ~SPI_MISO_PIN;
// Configure the RESET and DIO0 pins as outputs
P1DIR |= RESET_PIN | DIO0_PIN;
// Configure the UART pins for SPI communication
P1SEL |= SPI_CLK_PIN | SPI_MISO_PIN | SPI_MOSI_PIN;
P1SEL2 |= SPI_CLK_PIN | SPI_MISO_PIN | SPI_MOSI_PIN;
// Configure the USCI_B0 module for SPI communication
UCB0CTL1 |= UCSWRST; // Reset the module
UCB0CTL0 = UCCKPH | UCMSB | UCMST | UCSYNC; // Configure the SPI settings
UCB0CTL1 = UCSSEL_2 | UCSWRST; // Configure the clock source for SMCLK
UCB0BR0 = 2; // Set the clock divider to 2
UCB0BR1 = 0;
UCB0CTL1 &= ~UCSWRST; // Release the module from reset
// Reset the LoRa module
LoRa_Reset();
// Configure the LoRa module
SPI_Write(0x01, 0x80); // Sleep mode
SPI_Write(0x01, 0x81); // Standby mode
SPI_Write(0x06, 0x6C); // Set the frequency to 915MHz
SPI_Write(0x07, 0x00); // Set the frequency deviation to 0
SPI_Write(0x0D, 0x00); // Set the spreading factor to 6
SPI_Write(0x0E, 0x08); // Set the bandwidth to 125kHz
SPI_Write(0x0F, 0x0A); // Set the coding rate to 4/5
SPI_Write(0x1D, 0x72); // Enable the CRC check
SPI_Write(0x1E, 0x08); // Set the payload length to 8 bytes
SPI_Write(0x26, 0x07); // Set the preamble length to 7 bytes
SPI_Write(0x12, 0xFF); // Set the maximum output power
}
void main(void)
{
WDTCTL = WDTPW + WDTHOLD; // Disable the watchdog timer
LoRa_Init(); // Initialize the LoRa module
while(1)
{
uint8_t data[] = {0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08};
SPI_Write(0x0A, 0x80); // Set the module to transmit mode
SPI_Write(0x0D, 0x0C); // Set the spreading factor to 12
SPI_Write(0x0E, 0x08); // Set the bandwidth to 125kHz
SPI_Write(0x1D, 0x00); // Disable the CRC check
SPI_Write(0x1E, sizeof(data)); // Set the payload length to the size of the data
for(uint8_t i = 0; i < sizeof(data); i++)
{
SPI_Write(0x00, data[i]); // Write the data to the FIFO
}
SPI_Write(0x0F, 0x0A); // Set the coding rate to 4/5
SPI_Write(0x26, 0x07); // Set the preamble length to 7 bytes
SPI_Write(0x01, 0x83); // Transmit mode
while(!(P1IN & DIO0_PIN)); // Wait for DIO0 to go high
SPI_Read(0x12); // Read the IRQ flags to clear them
SPI_Write(0x01, 0x81); // Standby mode
__delay_cycles(1000000); // Wait for 1s
}
}
```
这段代码使用 MSP430 的 USCI_B0 模块进行 SPI 通信,来与 LoRa 模块进行数据的发送和接收。在这个示例中,我们将模块配置为发送模式,并发送一个长度为 8 字节的数据包。在发送完成后,我们等待接收到 LoRa 模块的中断信号(通过 DIO0 引脚),然后清除 IRQ 标志并进入待机模式,等待下一次发送。请注意,这只是一个简单的示例,实际应用中可能需要更复杂的配置和处理逻辑。
相关推荐
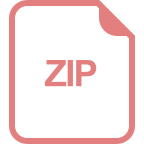














