帮我写一个stm32的串口函数
时间: 2024-05-10 07:21:23 浏览: 13
以下是一个简单的STM32串口函数示例,使用HAL库和DMA传输:
```c
#include "stm32f4xx_hal.h"
#include <string.h>
#define UART_TX_BUFFER_SIZE 128
#define UART_RX_BUFFER_SIZE 128
UART_HandleTypeDef huart2;
DMA_HandleTypeDef hdma_usart2_rx;
DMA_HandleTypeDef hdma_usart2_tx;
uint8_t uart_tx_buffer[UART_TX_BUFFER_SIZE];
uint8_t uart_rx_buffer[UART_RX_BUFFER_SIZE];
// 初始化串口
void uart_init(void)
{
huart2.Instance = USART2;
huart2.Init.BaudRate = 115200;
huart2.Init.WordLength = UART_WORDLENGTH_8B;
huart2.Init.StopBits = UART_STOPBITS_1;
huart2.Init.Parity = UART_PARITY_NONE;
huart2.Init.Mode = UART_MODE_TX_RX;
huart2.Init.HwFlowCtl = UART_HWCONTROL_NONE;
huart2.Init.OverSampling = UART_OVERSAMPLING_16;
HAL_UART_Init(&huart2);
// 配置DMA传输
hdma_usart2_rx.Instance = DMA1_Stream5;
hdma_usart2_rx.Init.Channel = DMA_CHANNEL_4;
hdma_usart2_rx.Init.Direction = DMA_PERIPH_TO_MEMORY;
hdma_usart2_rx.Init.PeriphInc = DMA_PINC_DISABLE;
hdma_usart2_rx.Init.MemInc = DMA_MINC_ENABLE;
hdma_usart2_rx.Init.PeriphDataAlignment = DMA_PDATAALIGN_BYTE;
hdma_usart2_rx.Init.MemDataAlignment = DMA_MDATAALIGN_BYTE;
hdma_usart2_rx.Init.Mode = DMA_CIRCULAR;
hdma_usart2_rx.Init.Priority = DMA_PRIORITY_HIGH;
hdma_usart2_rx.Init.FIFOMode = DMA_FIFOMODE_DISABLE;
HAL_DMA_Init(&hdma_usart2_rx);
__HAL_LINKDMA(&huart2, hdmarx, hdma_usart2_rx);
hdma_usart2_tx.Instance = DMA1_Stream6;
hdma_usart2_tx.Init.Channel = DMA_CHANNEL_4;
hdma_usart2_tx.Init.Direction = DMA_MEMORY_TO_PERIPH;
hdma_usart2_tx.Init.PeriphInc = DMA_PINC_DISABLE;
hdma_usart2_tx.Init.MemInc = DMA_MINC_ENABLE;
hdma_usart2_tx.Init.PeriphDataAlignment = DMA_PDATAALIGN_BYTE;
hdma_usart2_tx.Init.MemDataAlignment = DMA_MDATAALIGN_BYTE;
hdma_usart2_tx.Init.Mode = DMA_NORMAL;
hdma_usart2_tx.Init.Priority = DMA_PRIORITY_HIGH;
hdma_usart2_tx.Init.FIFOMode = DMA_FIFOMODE_DISABLE;
HAL_DMA_Init(&hdma_usart2_tx);
__HAL_LINKDMA(&huart2, hdmatx, hdma_usart2_tx);
// 启动DMA传输
HAL_UART_Receive_DMA(&huart2, uart_rx_buffer, UART_RX_BUFFER_SIZE);
}
// 发送一串数据
void uart_send(const uint8_t *data, uint16_t length)
{
// 等待发送缓冲区空闲
while (huart2.gState != HAL_UART_STATE_READY) {}
// 复制数据到发送缓冲区
memcpy(uart_tx_buffer, data, length);
// 启动DMA传输
HAL_DMA_Start(&hdma_usart2_tx, (uint32_t)uart_tx_buffer, (uint32_t)&huart2.Instance->DR, length);
HAL_UART_Transmit_DMA(&huart2, uart_tx_buffer, length);
}
// 接收数据中断回调函数
void HAL_UART_RxCpltCallback(UART_HandleTypeDef *huart)
{
// 处理接收到的数据
// ...
}
// 发送数据中断回调函数
void HAL_UART_TxCpltCallback(UART_HandleTypeDef *huart)
{
// 发送完成
}
```
使用示例:
```c
int main(void)
{
HAL_Init();
uart_init();
uint8_t msg[] = "Hello, world!\r\n";
uart_send(msg, sizeof(msg));
while (1) {}
return 0;
}
```
相关推荐
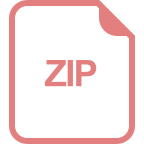














