java opencv矫正截取条形码
时间: 2024-08-29 12:01:18 浏览: 58
在Java中使用OpenCV矫正并截取条形码的过程通常包括以下几个步骤:
1. **图像预处理**:首先,需要对图像进行预处理,包括灰度化、二值化等操作,以便更好地识别条形码区域。
2. **边缘检测**:通过边缘检测算法(如Canny边缘检测)来找到图像中的边缘,这有助于识别条形码的轮廓。
3. **图像矫正**:利用透视变换(Perspective Transform)来矫正倾斜或扭曲的条形码图像,使得条形码区域尽可能水平且无扭曲。
4. **条形码区域定位**:根据矫正后的图像,可以通过轮廓检测找到条形码的具体位置和大小。
5. **条形码截取**:一旦定位到条形码区域,就可以将这部分图像从原图中截取出来。
6. **条形码解码**:最后,使用OpenCV中的条形码解码功能对截取的条形码图像进行解码,获取条形码中存储的信息。
以下是一个简化的代码示例,说明如何使用OpenCV进行条形码的矫正和截取:
```java
import org.opencv.core.Core;
import org.opencv.core.CvType;
import org.opencv.core.Mat;
import org.opencv.core.Point;
import org.opencv.core.Rect;
import org.opencv.core.Scalar;
import org.opencv.imgproc.Imgproc;
import org.opencv.calib3d.Calib3d;
// 加载OpenCV本地库
static {
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
}
public void extractBarcode(Mat src, Mat dst) {
// 将图像转换为灰度图
Mat gray = new Mat();
Imgproc.cvtColor(src, gray, Imgproc.COLOR_BGR2GRAY);
// 应用高斯模糊
Imgproc.GaussianBlur(gray, gray, new org.opencv.core.Size(5, 5), 0);
// 边缘检测
Mat edges = new Mat();
Imgproc.Canny(gray, edges, 75, 200);
// 找到轮廓
List<MatOfPoint> contours = new ArrayList<>();
Mat hierarchy = new Mat();
Imgproc.findContours(edges, contours, hierarchy, Imgproc.RETR_LIST, Imgproc.CHAIN_APPROX_SIMPLE);
// 遍历轮廓,找到最大的轮廓(假设是条形码)
MatOfPoint2f maxContour = new MatOfPoint2f();
double maxArea = 0;
for (MatOfPoint contour : contours) {
MatOfPoint2f contour2f = new MatOfPoint2f(contour.toArray());
double area = Imgproc.contourArea(contour);
if (area > maxArea) {
maxArea = area;
maxContour = contour2f;
}
}
// 如果找到了轮廓,则进行透视变换矫正
if (maxContour.rows() > 4) {
// 近似轮廓
MatOfPoint2f approxCurve = new MatOfPoint2f();
Imgproc.approxPolyDP(maxContour, approxCurve, Imgproc.arcLength(maxContour, true) * 0.02, true);
// 确定源点和目标点
Point[] srcPts = approxCurve.toArray();
Point[] dstPts = new Point[]{
new Point(0, 0), new Point(src.cols(), 0),
new Point(src.cols(), src.rows()), new Point(0, src.rows())
};
// 计算透视变换矩阵
Mat perspectiveMatrix = Calib3d.findHomography(new MatOfPoint2f(srcPts), new MatOfPoint2f(dstPts));
// 应用透视变换
Core.perspectiveTransform(maxContour, maxContour, perspectiveMatrix);
Core.perspectiveTransform(approxCurve, approxCurve, perspectiveMatrix);
// 计算边界矩形并截取条形码区域
Rect rect = Imgproc.boundingRect(approxCurve);
Rect rectD = new Rect(rect.x, rect.y, rect.width, rect.height);
Mat barcode = new Mat(src, rectD);
// 将矫正后的条形码复制到dst图像中
barcode.copyTo(dst);
} else {
// 如果未找到轮廓,则dst保持不变
}
}
// 使用示例
Mat srcImage = new Mat(...); // 加载你的图像
Mat dstImage = new Mat(srcImage.size(), CvType.CV_8UC3);
extractBarcode(srcImage, dstImage);
```
阅读全文
相关推荐
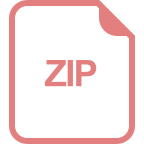
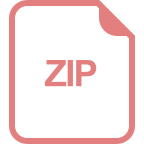

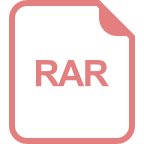


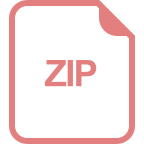
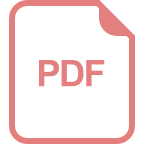
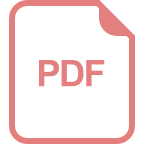
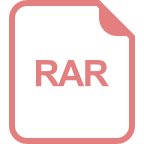
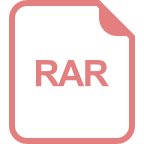
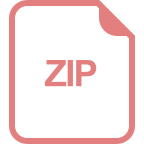
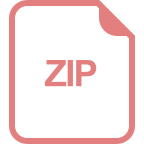
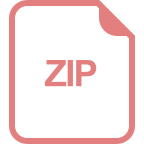