c++ 将float转为字符串
时间: 2024-04-19 12:19:03 浏览: 196
在C++中,有多种方法可以将float类型转换为string类型。以下是其中的三种方法:
方法一:
```c++
#include <sstream>
std::string Convert(float Num) {
std::ostringstream oss;
oss << Num;
std::string str(oss.str());
return str;
}
```
方法二:
```c++
#include <sstream>
int main(){
float num = 10.11;
std::string data = std::to_string(num);
printf("data: %s\n", data.c_str());
return 0;
}
```
方法三:
```c++
#include <cstdio>
#include <cstring>
#include <cmath>
#include <cstdlib>
#include <iostream>
using namespace std;
string FloatToString(float f) {
char buf[32];
sprintf(buf, "%.2f", f);
return string(buf);
}
```
以上三种方法都可以将float类型转换为string类型,具体使用哪种方法取决于你的需求和个人喜好。
相关问题
C++如何将数字转为字符串
在C中,可以使用sprintf()函数将数字转换为字符串。
例如,以下代码将整数num转换为字符串str:
```c
int num = 123;
char str[10];
sprintf(str, "%d", num);
```
如果要将浮点数转换为字符串,则可以使用以下代码:
```c
float num = 3.14;
char str[10];
sprintf(str, "%.2f", num);
```
在上面的代码中,"%.2f"表示将浮点数num格式化为两位小数的字符串。
c++将字符串转为浮点数
可以使用C++标准库中的stof函数将字符串转换为浮点数。函数原型如下:
```c++
float stof(const string& str, size_t* idx = 0);
```
其中,str是待转换的字符串,idx表示从哪个位置开始转换,默认为0。
下面是一个示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str = "3.1415926";
float f = stof(str);
cout << "f = " << f << endl;
return 0;
}
```
输出结果为:
```c++
f = 3.14159
```
注意,如果转换的字符串不是合法的浮点数格式,将会抛出std::invalid_argument异常。
阅读全文
相关推荐
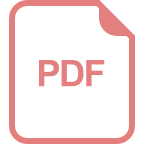
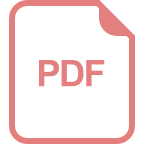





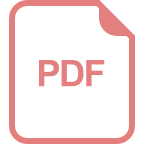
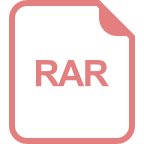




