用c语言写一个:输入一行字符,分别统计出其中英文字母、空格、数字和其他字符的个数。
时间: 2023-09-16 18:05:42 浏览: 181
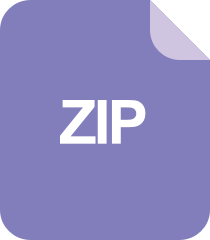
输入一行字符,分别统计出其中的英文字母、空格、数字、和其他字符个数
### 回答1:
下面是一个在 C 语言中实现上述功能的代码示例:
```
#include <stdio.h>
#include <ctype.h>
int main()
{
char c;
int letters = 0, spaces = 0, digits = 0, others = 0;
printf("请输入一行字符:");
while ((c = getchar()) != '\n')
{
if (isalpha(c))
{
letters++;
}
else if (isspace(c))
{
spaces++;
}
else if (isdigit(c))
{
digits++;
}
else
{
others++;
}
}
printf("字母个数:%d\n", letters);
printf("空格个数:%d\n", spaces);
### 回答2:
这是一个简单的C语言程序,用于统计输入一行字符中英文字母、空格、数字和其他字符的个数:
```c
#include <stdio.h>
int main() {
char input[100];
int i;
int alpha_count = 0;
int space_count = 0;
int digit_count = 0;
int other_count = 0;
printf("请输入一行字符:");
fgets(input, sizeof(input), stdin);
for (i = 0; input[i] != '\0'; i++) {
if ((input[i] >= 'a' && input[i] <= 'z') || (input[i] >= 'A' && input[i] <= 'Z')) {
alpha_count++; // 统计英文字母个数
}
else if (input[i] == ' ') {
space_count++; // 统计空格个数
}
else if (input[i] >= '0' && input[i] <= '9') {
digit_count++; // 统计数字个数
}
else {
other_count++; // 统计其他字符个数
}
}
printf("英文字母个数:%d\n", alpha_count);
printf("空格个数:%d\n", space_count);
printf("数字个数:%d\n", digit_count);
printf("其他字符个数:%d\n", other_count);
return 0;
}
```
在运行程序时,会提示用户输入一行字符,然后根据输入的字符统计英文字母、空格、数字和其他字符的个数,并将结果打印出来。
### 回答3:
可以使用C语言编写一个程序来实现输入一行字符,并分别统计其中的英文字母、空格、数字和其他字符的个数。以下为一个简单的示例代码:
```c
#include <stdio.h>
int main() {
char input[100]; // 假设输入的字符不会超过100个
int i, alphabets = 0, spaces = 0, digits = 0, others = 0;
printf("请输入一行字符: ");
fgets(input, sizeof(input), stdin); // 从标准输入中获取一行字符
for (i = 0; input[i] != '\0'; i++) {
if ((input[i] >= 'a' && input[i] <= 'z') || (input[i] >= 'A' && input[i] <= 'Z')) {
alphabets++;
} else if (input[i] == ' ') {
spaces++;
} else if (input[i] >= '0' && input[i] <= '9') {
digits++;
} else {
others++;
}
}
printf("英文字母个数: %d\n", alphabets);
printf("空格个数: %d\n", spaces);
printf("数字个数: %d\n", digits);
printf("其他字符个数: %d\n", others);
return 0;
}
```
该程序首先定义了一个字符数组 `input[]` 来存储输入的字符,然后使用 `fgets()` 从标准输入中获取一行字符。接下来,通过 `for` 循环遍历每个字符,并进行相应的判断和统计。最后,输出统计结果。
注意:上述实现中,我们使用了 ASCII 码的值范围来判断字符是否属于英文字母、数字或其他字符。若需要考虑其它字符集,请适当修改判断条件。
阅读全文
相关推荐















